How to Test Chef Code using InSpec
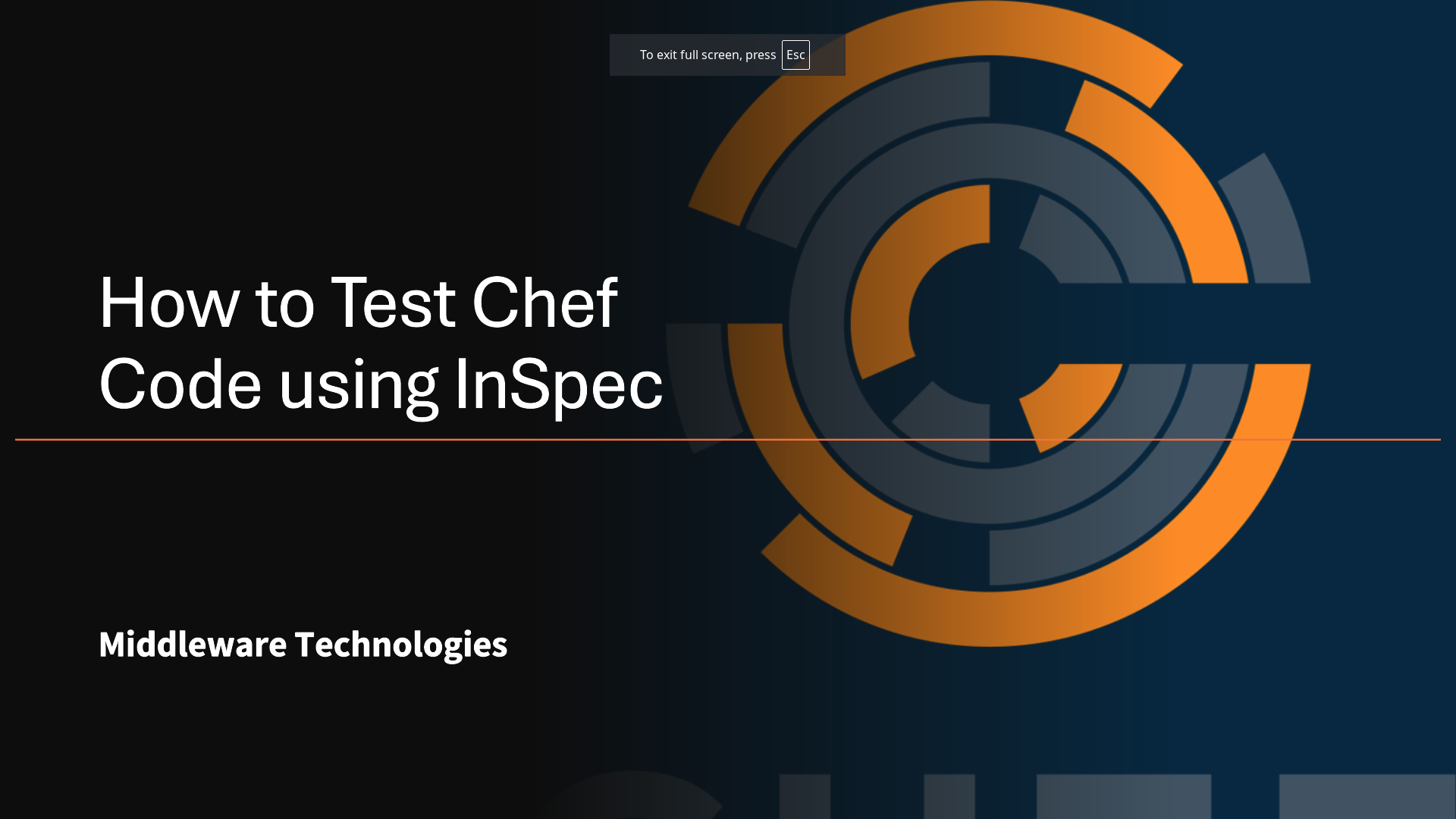
Here in this article, we will see how we can validate or test the Chef Infrastructure code using InSpec, which we develop for managing or maintaining our systems in the desired state.
Test Environment
Workstation with ChefDK
Virtualbox
Vagrant
Procedure
Step1: Checking the Installed Versions of Chef and related tools
Validate the ChefDK version installed on your system.
[root@desktop1 ~]# chef --version
Chef Development Kit Version:
2.0.26
chef-client version: 13.2.20
delivery version: master
berks version: 6.2.0
kitchen version: 1.16.0
inspec version: 1.30.0
[root@desktop1 ~]# docker
--version
Docker version 17.03.2-ce,
build f5ec1e2
[root@desktop1 ~]# vagrant --version
Vagrant 1.9.6
Step2: Generate Cookbook
Here we will generate a sample cookbook named “webserver_test” as shown below.
[root@desktop1 cookbooks]# pwd
/root/stack/middleware/chefspace/cookbooks
[root@desktop1 cookbooks]# chef generate cookbook webserver_test
Generating cookbook webserver_test
- Ensuring correct cookbook file content
- Committing cookbook files to git
- Ensuring delivery configuration
- Ensuring correct delivery build cookbook content
- Adding delivery configuration to feature branch
- Adding build cookbook to feature branch
- Merging delivery content feature branch to master
Your cookbook is ready. Type `cd webserver_test` to enter it.
There are several commands you can run to get started locally developing and
testing your cookbook.
Type `delivery local --help` to see a full list.
Why not start by writing a test? Tests for the default recipe are stored at:
test/smoke/default/default_test.rb
If you'd prefer to dive right in, the default recipe can be found at:
recipes/default.rb
Step3: Update the .kitchen.yml with the appropriate Drivers and Image details as below
Let’s now update the kitchen.yml file with image that we want to test upon and required drivers for instantiating the virtual environment.
[root@desktop1 webserver_test]# cat .kitchen.yml
---
driver:
name: vagrant
provider: docker
provisioner:
name: chef_zero
# You may wish to disable always updating cookbooks in
# CI or other testing environments.
# For example:
# always_update_cookbooks: <%= !ENV['CI'] %>
# always_update_cookbooks: true
verifier:
name: inspec
platforms:
- name: tknerr/baseimage-ubuntu-14.04
suites:
- name: default
run_list:
- recipe[webserver_test::default]
verifier:
inspec_tests:
- test/smoke/default
attributes:
Step4: Update the default_test.rb file with InSpec code for validating the webserver functionality
Now let’s update our test Inspec code as shown below to test our service status and check the application page.
[root@desktop1 webserver_test]# cat test/smoke/default/default_test.rb
# # encoding: utf-8
# Inspec test for recipe webserver_test::default
# The Inspec reference, with examples and extensive documentation, can be
# found at http://inspec.io/docs/reference/resources/
describe package('apache2') do
it {should be_installed}
end
describe service('apache2') do
it {should be_enabled}
it {should be_running}
end
describe command('curl localhost') do
its('stdout') {should match /hello/}
end
describe port(80) do
it {should be_listening}
end
Step5: Update the default.rb recipe with the Infrastructure code for installing and configuring a basic webserver
Here is our updated recipe to install and configure our webserver as per the testing requirements that we defined in our InSpec code.
[root@desktop1 webserver_test]# cat recipes/default.rb
#
# Cookbook:: webserver_test
# Recipe:: default
#
# Copyright:: 2017, The Authors, All Rights Reserved.
apt_update 'update' do
action :update
end
package 'apache2'
service 'apache2' do
action [:enable,:start]
end
file '/var/www/html/index.html' do
content '
hello world
'
end
Step6: Test the Chef Code in a Test Kitchen using InSpec
Now its time to test our setup using “kitchen test” as shown below.
[root@desktop1 webserver_test]# kitchen test
-----> Starting Kitchen (v1.16.0)
-----> Cleaning up any prior instances of
-----> Destroying ...
==> default: Stopping container...
==> default: Deleting the container...
Vagrant instance destroyed.
Finished destroying (0m9.73s).
-----> Testing
...
* apt_package[apache2] action install
- install version 2.4.7-1ubuntu4.16 of package apache2
* service[apache2] action enable (up to date)
* service[apache2] action start
- start service service[apache2]
* file[/var/www/html/index.html] action create
- update content in file /var/www/html/index.html from 538f31 to e53067
--- /var/www/html/index.html 2017-07-10 09:31:02.697553595 +0000
+++ /var/www/html/.chef-index20170710-195-30i74n.html
2017-07-10 09:31:10.261551246 +0000
@@ -1,379 +1,7 @@
Note – Kitchen test is going to internally destroy any containers if present,
create a test kitchen, converge the infrastructure code, verify the code and
finally destroy the container instance.
kitchen destroy
kitchen create
kitchen converge
kitchen verify
kitchen destroy
Hope you enjoyed reading this article. Thank you..
Leave a Reply
You must be logged in to post a comment.