How to setup AWS Lambda Function using AWS CLI
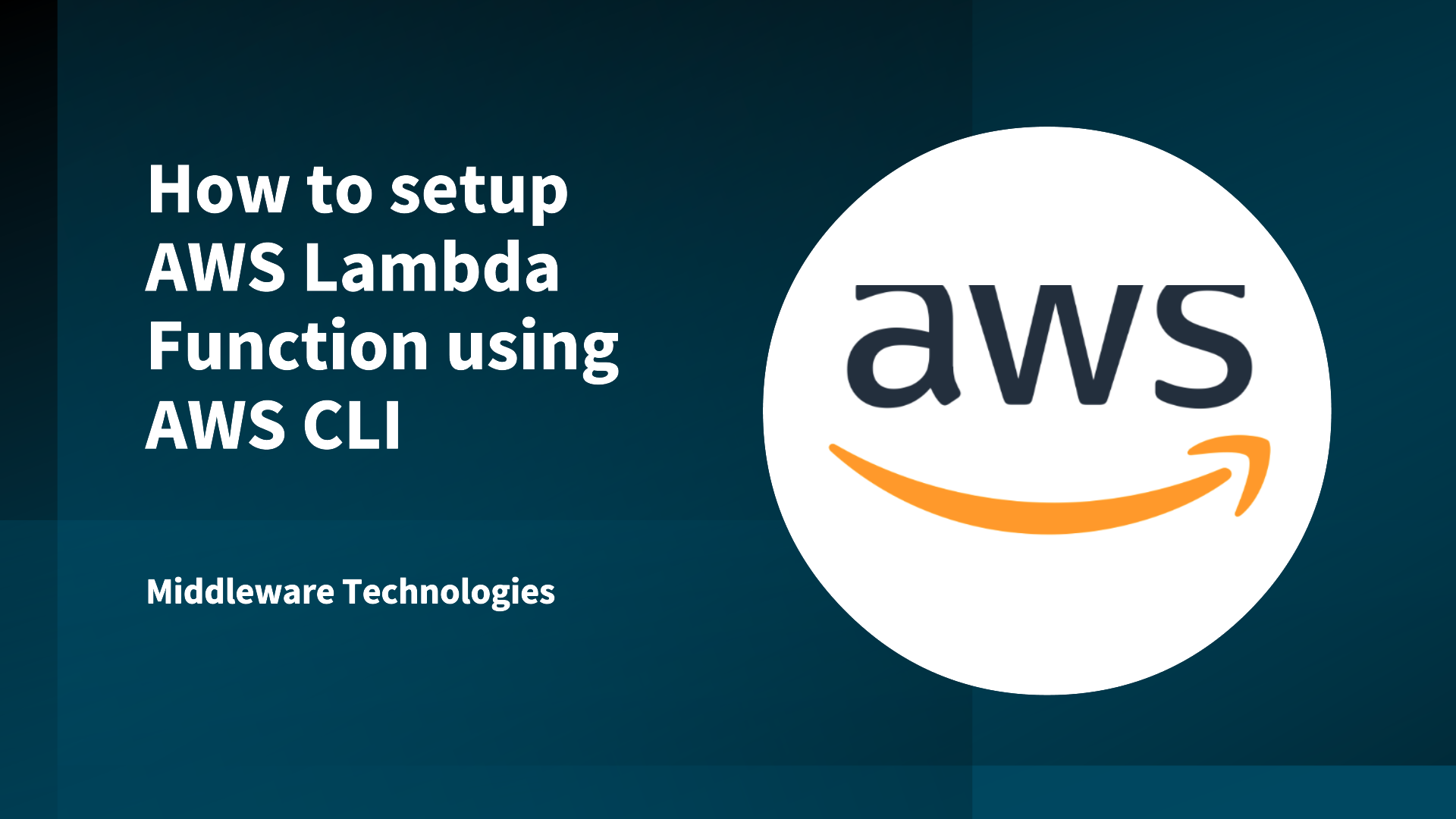
Here in this article we will try to setup a sample AWS Lambda function based on python language using the AWS CLI commands and validate its execution.
Test Environment
AWS Cloud
What is AWS Lambda
AWS Lambda is a serverless feature provided by AWS Cloud. It can be used to run code without provisioning or managing servers. The only that is needs to be supplied to AWS lambda service is code in one supported language runtime. Lambda is an ideal compute service for application scenarios that need to scale up rapidly, and scale down to zero when not in demand.
AWS Lambda Benefits
- Highly available compute infrastructure
- Managed Server and OS environment
- Automatic Scaling and Provisiong of resources
- Logging
NOTE: We assume you have access to AWS Cloud and have a user with Administrative access already created and AWS CLI configured on your workstation.
If you are interested in watching the video. Here is the YouTube video on the same step by step procedure outlined below.
Procedure
Step1: Create and Package lambda function code
Here we will create a python lambda_function.py file which will be used to calculate the area of an object with length and width parameters.
- Lambda Handler: The lambda_handler function is the entry point into a lambda function code. When your function is invoked, Lambda runs this method.
- Lambda event object: An event in Lambda is a JSON formatted document that contains data for your function to process.
- Lambda context object: The context object contains information about the function invocation and execution environment.
$ cat lambda_function.py
import json
import logging
logger = logging.getLogger()
logger.setLevel(logging.INFO)
def lambda_handler(event, context):
# Get the length and width parameters from the event object. The
# runtime converts the event object to a Python dictionary
length = event['length']
width = event['width']
area = calculate_area(length, width)
print(f"The area is {area}")
logger.info(f"CloudWatch logs group: {context.log_group_name}")
# return the calculated area as a JSON string
data = {"area": area}
return json.dumps(data)
def calculate_area(length, width):
return length*width
Once our code is ready we can zip our function code as shown below.
$ zip lambda_function.zip lambda_function.py
This will create a zip file as shown below.
-rw-r--r--. 1 admin admin 523 Jan 19 17:57 lambda_function.zip
Step2: Create a Execution role
The trust policy defines which principals can assume the role, and under which conditions. A trust policy is a specific type of resource-based policy for IAM roles.
File: trustpolicyforlambdaservice.json
{
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Action": [
"sts:AssumeRole"
],
"Principal": {
"Service": [
"lambda.amazonaws.com"
]
}
}
]
}
Let’s create a role named “MyTestFunction-role” with the above trust policy document as shown below.
$ aws iam create-role --role-name MyTestFunction-role --assume-role-policy-document file://trustpolicyforlambdaservice.json
{
"Role": {
"Path": "/",
"RoleName": "MyTestFunction-role",
"RoleId": "AROASV7XIM3SNVY4LRVZT",
"Arn": "arn:aws:iam::xxx:role/MyTestFunction-role",
"CreateDate": "2025-01-20T12:40:28+00:00",
"AssumeRolePolicyDocument": {
"Version": "2012-10-17",
"Statement": [
{
"Effect": "Allow",
"Action": [
"sts:AssumeRole"
],
"Principal": {
"Service": [
"lambda.amazonaws.com"
]
}
}
]
}
}
}
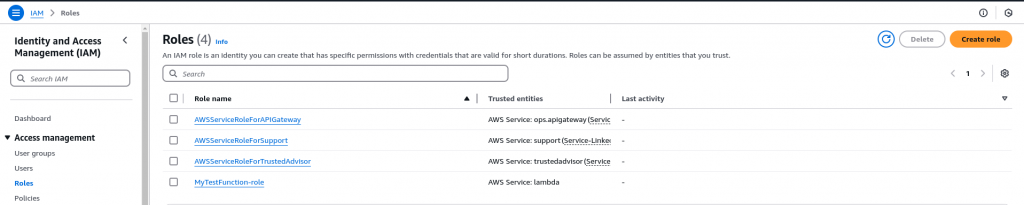
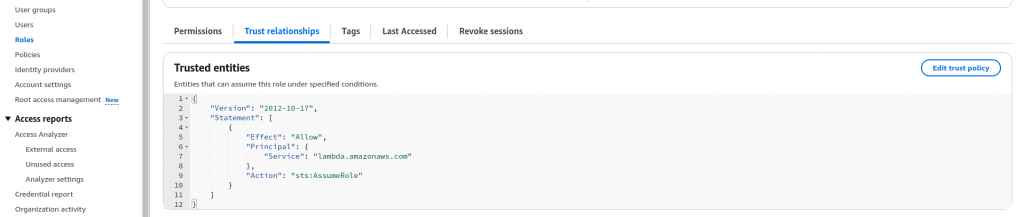
Here we are attaching the managed role policy named “AWSLambdaBasicExecutionRole” with the IAM role “MyTestFunction-role”.
$ aws iam attach-role-policy --role-name MyTestFunction-role --policy-arn arn:aws:iam::aws:policy/service-role/AWSLambdaBasicExecutionRole

Step3: Create a AWS Lambda function
Now it’s time to create our lambda function using the below cli command with the following options shown below. Please update the role arn with the correct value. Update “xxx” with your account id.
$ aws lambda create-function \
--function-name my-function \
--runtime python3.13 \
--zip-file fileb://lambda_function.zip \
--handler lambda_function.lambda_handler \
--role arn:aws:iam::xxx:role/MyTestFunction-role
Output:
{
"FunctionName": "my-function",
"FunctionArn": "arn:aws:lambda:us-east-1:xxx:function:my-function",
"Runtime": "python3.13",
"Role": "arn:aws:iam::xxx:role/MyTestFunction-role",
"Handler": "lambda_function.lambda_handler",
"CodeSize": 523,
"Description": "",
"Timeout": 3,
"MemorySize": 128,
"LastModified": "2025-01-20T12:41:09.264+0000",
"CodeSha256": "0VFDHIZd9oLP/wgCszOWvdraTpmQceXag6lW+2PDCss=",
"Version": "$LATEST",
"TracingConfig": {
"Mode": "PassThrough"
},
"RevisionId": "9f6180ae-710c-48f1-a682-6e265c76ab60",
"State": "Pending",
"StateReason": "The function is being created.",
"StateReasonCode": "Creating",
"PackageType": "Zip",
"Architectures": [
"x86_64"
]
}

Step4: Invoke Lambda function
We can invoke a function synchronously (and wait for the response), or asynchronously. By default, Lambda invokes your function synchronously (i.e. the“InvocationType“ is RequestResponse ). To invoke a function asynchronously, set InvocationType to Event . Lambda passes the ClientContext object to your function for synchronous invocations only.
Asynchronous invoke
$ aws lambda invoke \
--function-name my-function \
--invocation-type Event \
--cli-binary-format raw-in-base64-out \
--payload '{ "length": 6, "width": 7}' \
--output json \
response.json
Synchronous invoke
$ aws lambda invoke \
--function-name my-function \
--cli-binary-format raw-in-base64-out \
--payload '{ "length": 6, "width": 7}' \
--output json \
response.json
{
"StatusCode": 200,
"ExecutedVersion": "$LATEST"
}
Output:
$ cat response.json
"{\"area\": 42}"
You can also look at the function cloudformation template that gets generated automatically in the AWS console by Navigating to your function and viewing the template. Please read the comments below before using this template for automation.
# This AWS SAM template has been generated from your function's configuration. If
# your function has one or more triggers, note that the AWS resources associated
# with these triggers aren't fully specified in this template and include
# placeholder values. Open this template in AWS Infrastructure Composer or your
# favorite IDE and modify it to specify a serverless application with other AWS
# resources.
AWSTemplateFormatVersion: '2010-09-09'
Transform: AWS::Serverless-2016-10-31
Description: An AWS Serverless Application Model template describing your function.
Resources:
myfunction:
Type: AWS::Serverless::Function
Properties:
CodeUri: .
Description: ''
MemorySize: 128
Timeout: 3
Handler: lambda_function.lambda_handler
Runtime: python3.13
Architectures:
- x86_64
EphemeralStorage:
Size: 512
EventInvokeConfig:
MaximumEventAgeInSeconds: 21600
MaximumRetryAttempts: 2
PackageType: Zip
Policies:
- Statement:
- Effect: Allow
Action:
- logs:CreateLogGroup
- logs:CreateLogStream
- logs:PutLogEvents
Resource: '*'
RecursiveLoop: Terminate
SnapStart:
ApplyOn: None
RuntimeManagementConfig:
UpdateRuntimeOn: Auto
Hope you enjoyed reading this article. Thank you..
Leave a Reply
You must be logged in to post a comment.