How to use OWASP Dependency-Check for third party libraries scan
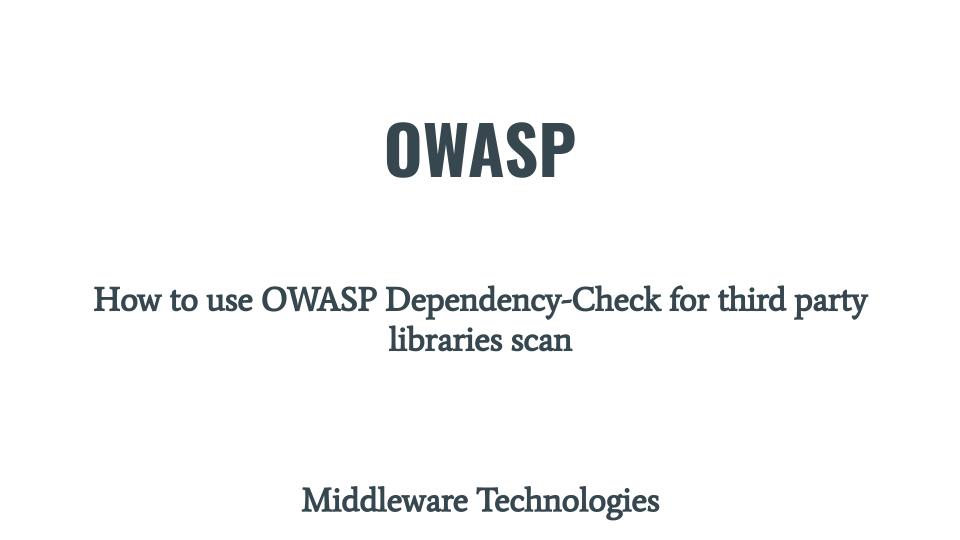
Here in this article we will be using a sample java spring boot based application and update the project with OWASP dependency-check-gradle plugin which will help us in scanning all the dependent libraries for any vulnerabilities and generate a report.
What is OWASP Dependency-Check
As per OWASP documentation, Dependency-Check is a Software Composition Analysis (SCA) tool that attempts to detect publicly disclosed vulnerabilities contained within a project’s dependencies. It does this by determining if there is a Common Platform Enumeration (CPE) identifier for a given dependency. If found, it will generate a report linking to the associated CVE entries.
If you are interested in watching the video. Here is the YouTube video on the same step by step procedure outlined below.
Procedure
Step1: Request NVD API key
OWASP dependency check tool uses the National Vulnerability Database for the information on the CVE’s related to different software components or entities. This is an optional step to request for an API key to use the NVD database. In case we are not using the API key the rate at which we will be able to download the NVD data would be slow. For this reason its recommended to generate an API key using Request an API Key.
Once you submit the required details you will receive an email with a link to generate your personal API key which need to be secured.
Step2: Create a Java application
Here in this step i am using vscode to generate a sample hello world java application based on the gradle build. The structure of the project is as below.
admin@fedser:gradledepcheck$ tree .
.
├── app
│ ├── build.gradle
│ └── src
│ ├── main
│ │ ├── java
│ │ │ └── gradledepcheck
│ │ │ └── App.java
│ │ └── resources
│ └── test
│ ├── java
│ │ └── gradledepcheck
│ │ └── AppTest.java
│ └── resources
├── gradle
│ └── wrapper
│ ├── gradle-wrapper.jar
│ └── gradle-wrapper.properties
├── gradlew
├── gradlew.bat
└── settings.gradle
Step3: Apply Dependency Check Plugin
In this step we are going to update our build.gradle for the sample project by applying OWASP dependency check plugin as shown below. This plugin provides us with different tasks which will help us in fetching the vulnerability database and also scan projects for any vulnerabilities based on the available data.
admin@fedser:gradledepcheck$ cat app/build.gradle
/*
* This file was generated by the Gradle 'init' task.
*
* This generated file contains a sample Java application project to get you started.
* For more details take a look at the 'Building Java & JVM projects' chapter in the Gradle
* User Manual available at https://docs.gradle.org/8.0.2/userguide/building_java_projects.html
*/
plugins {
// Apply the application plugin to add support for building a CLI application in Java.
id 'application'
id "org.owasp.dependencycheck" version "9.0.9"
}
repositories {
// Use Maven Central for resolving dependencies.
mavenCentral()
}
dependencies {
// Use JUnit Jupiter for testing.
testImplementation 'org.junit.jupiter:junit-jupiter:5.9.1'
// This dependency is used by the application.
implementation 'com.google.guava:guava:31.1-jre'
}
application {
// Define the main class for the application.
mainClass = 'gradledepcheck.App'
}
tasks.named('test') {
// Use JUnit Platform for unit tests.
useJUnitPlatform()
}
dependencyCheck {
nvd {
apiKey='xxxxxxxx'
}
}
Step4: Analyze Project
Once the plugin is applied we list the tasks that are provided by this plugin as shown below. As you can see it provides us with four different task for our usage.
admin@fedser:gradledepcheck$ ./gradlew tasks
...
OWASP dependency-check tasks
----------------------------
dependencyCheckAggregate - Identifies and reports known vulnerabilities (CVEs) in multi-project dependencies.
dependencyCheckAnalyze - Identifies and reports known vulnerabilities (CVEs) in project dependencies.
dependencyCheckPurge - Purges the local cache of the NVD.
dependencyCheckUpdate - Downloads and stores updates from the NVD CVE data feeds.
...
We will be using the “dependencyCheckAnalyze” task to scan the project to identify any vulnerabilties in the third party libraries that are being used by the project.
admin@fedser:gradledepcheck$ ./gradlew dependencyCheckAnalyze
> Task :app:dependencyCheckAnalyze
Verifying dependencies for project app
Checking for updates and analyzing dependencies for vulnerabilities
Generating report for project app
Found 2 vulnerabilities in project app
One or more dependencies were identified with known vulnerabilities in app:
guava-31.1-jre.jar (pkg:maven/com.google.guava/guava@31.1-jre, cpe:2.3:a:google:guava:31.1:*:*:*:*:*:*:*) : CVE-2023-2976, CVE-2020-8908
See the dependency-check report for more details.
Region [NODEAUDIT] : Not alive and dispose was called, filename: NODEAUDIT
Region [CENTRAL] : Not alive and dispose was called, filename: CENTRAL
Region [POM] : Not alive and dispose was called, filename: POM
BUILD SUCCESSFUL in 3s
1 actionable task: 1 executed
As you can see from the output, the build analysis has identifies two vulnerabilities in the dependent library jar “guava-31.1-jre.jar” used by the project. This analysis also generates a report for all the vulnerabilities that are identified within the project at the following build output location.
admin@fedser:gradledepcheck$ ls -ltr app/build/reports/
total 232
-rw-r--r--. 1 admin admin 44750 Mar 10 19:26 dependency-check-report.json
-rw-r--r--. 1 admin admin 189929 Mar 10 19:28 dependency-check-report.html
Step5: Break Build
In order to ensure that our build breaks whenever there is a vulnerability of a particular CVSS score is found in our project, we can update the build.gradle as shown below.
Here we are updating the file with “dependencyCheck” configuration to fail the build in case an vulnerability with a CVSS score of 7 or more is found. Also we want to ensure that the generated report in of JSON format. You can find more details about the configuration options at dependency check gradle configuration.
admin@fedser:gradledepcheck$ cat app/build.gradle
/*
* This file was generated by the Gradle 'init' task.
*
* This generated file contains a sample Java application project to get you started.
* For more details take a look at the 'Building Java & JVM projects' chapter in the Gradle
* User Manual available at https://docs.gradle.org/8.0.2/userguide/building_java_projects.html
*/
plugins {
// Apply the application plugin to add support for building a CLI application in Java.
id 'application'
id "org.owasp.dependencycheck" version "9.0.9"
}
repositories {
// Use Maven Central for resolving dependencies.
mavenCentral()
}
dependencies {
// Use JUnit Jupiter for testing.
testImplementation 'org.junit.jupiter:junit-jupiter:5.9.1'
// This dependency is used by the application.
implementation 'com.google.guava:guava:31.1-jre'
}
application {
// Define the main class for the application.
mainClass = 'gradledepcheck.App'
}
tasks.named('test') {
// Use JUnit Platform for unit tests.
useJUnitPlatform()
}
dependencyCheck {
failBuildOnCVSS=7
format='JSON'
nvd {
apiKey='xxxxxxxx'
}
}
Now let us try to run the analysis for our project and see if it breaks our build as per our requirement. If you have a CICD pipeline to build a project the following step can be introduced into the build to ensure that build wont proceed to next when a severity of a particular CVSS score is found in the project.
admin@fedser:gradledepcheck$ ./gradlew dependencyCheckAnalyze
Region [NODEAUDIT] : Not alive and dispose was called, filename: NODEAUDIT
Region [CENTRAL] : Not alive and dispose was called, filename: CENTRAL
Region [POM] : Not alive and dispose was called, filename: POM
> Task :app:dependencyCheckAnalyze FAILED
Verifying dependencies for project app
Checking for updates and analyzing dependencies for vulnerabilities
Generating report for project app
Found 2 vulnerabilities in project app
One or more dependencies were identified with known vulnerabilities in app:
guava-31.1-jre.jar (pkg:maven/com.google.guava/guava@31.1-jre, cpe:2.3:a:google:guava:31.1:*:*:*:*:*:*:*) : CVE-2023-2976, CVE-2020-8908
See the dependency-check report for more details.
FAILURE: Build failed with an exception.
* What went wrong:
Execution failed for task ':app:dependencyCheckAnalyze'.
>
Dependency-Analyze Failure:
One or more dependencies were identified with vulnerabilities that have a CVSS score greater than '7.0': CVE-2023-2976
See the dependency-check report for more details.
* Try:
> Run with --stacktrace option to get the stack trace.
> Run with --info or --debug option to get more log output.
> Run with --scan to get full insights.
* Get more help at https://help.gradle.org
BUILD FAILED in 2s
1 actionable task: 1 executed
Hope you enjoyed reading this article. Thank you..
Leave a Reply
You must be logged in to post a comment.