How to encrypt and use secrets using GitHub Actions
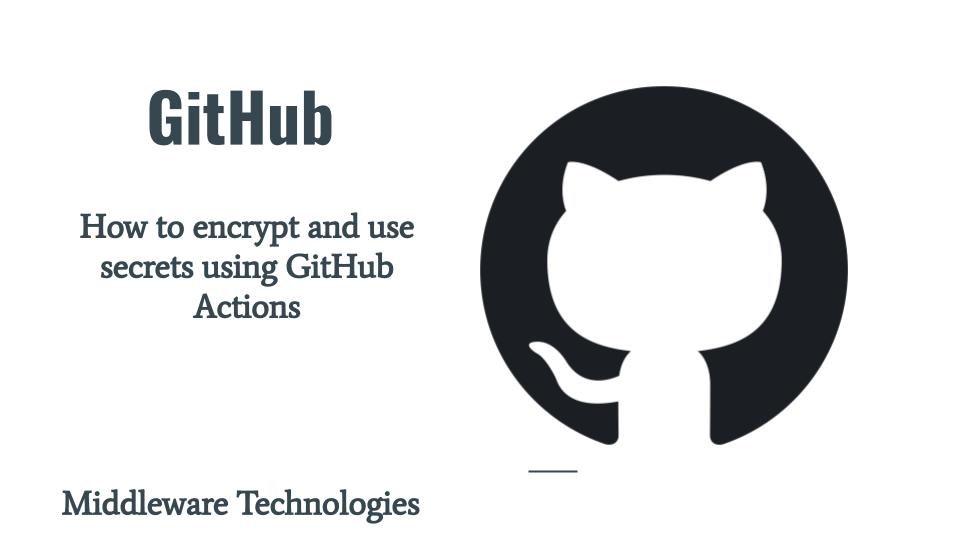
Here in this article we will try to see how we can encrypt a secret API key and token using GitHub secrets. We will further use these encrypted secrets to query the API to fetch the results.
Test Environment
Ubuntu 22.04
What are GitHub Actions Secrets
GitHub Actions Secrets provides us with a facility to store sensitive data like passwords, api keys, tokens and private keys using secrets. These secrets can be created and stored at the organization, repository or environment levels as per your requirements and can be used further in your workflows without exposing their values.
If you are interested in watching the video. Here is the YouTube video on the same step by step procedure outlined below.
Procedure
Step1: Clone Repository
As a first we are cloning a sample GitHub repository from my GitHub Account. You can fork this repository or create your own repository and use it for this activity.
[admin@fedser github_space]$ git clone https://github.com/novicejava1/learngit.git
Step2: Encrypt Secrets
Here in this step we are going to encrypt the api key and token for one of the api service. This is a service from rapidapi portal which is a compatibility calculator.
Go to Repository Settings – Secrets and variables – Actions. Under Secrets we will now create two “New repository secret” by providing the name and value. Here in this case it will your API access key and token.
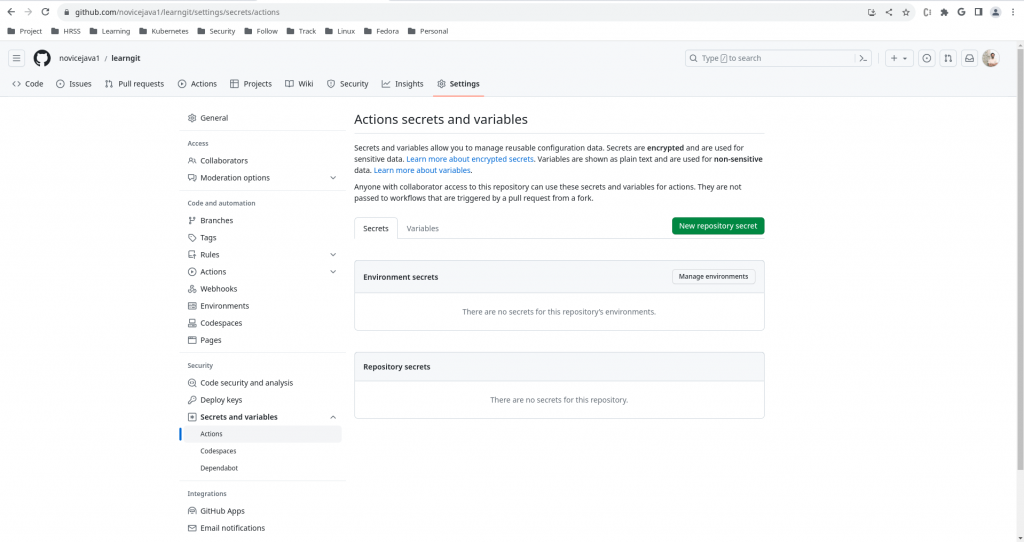
Once the secrets are added you can get to see the list of secrets as shown below.
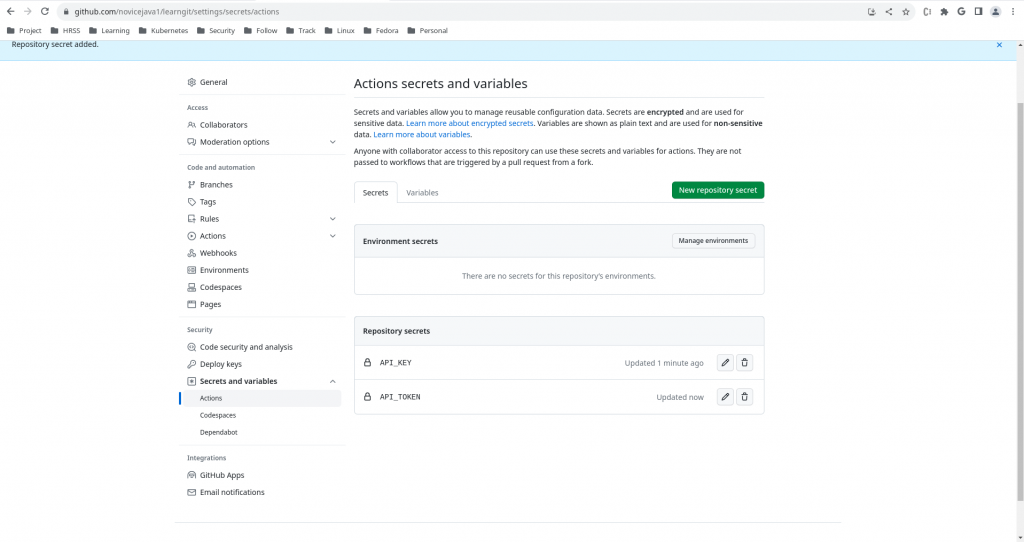
Step3: Create Python Script
Now let’s create a python script which will invoke this API service by utilizing the secrets that we just created. We are going to pass the API key and token as input arguments to the script.
[admin@fedser learngit]$ cat lovecal.py
import sys
import requests
api_key = sys.argv[1]
api_token = sys.argv[2]
def calculator(firstname, secondname):
url = "https://" + api_key + "/getPercentage"
querystring = {"fname": firstname,"sname": secondname}
headers = {
'x-rapidapi-host': api_key,
'x-rapidapi-key': api_token
}
response = requests.request("GET", url, headers=headers, params=querystring)
jsondata = response.json()
return jsondata
if __name__ == '__main__':
data = calculator("Adam", "Eve")
print(data)
Step4: Create Workflow
Here we will now create the workflow “apisecret.yml” which will checkout the repository, install python and dependent packages. In the last step we are going to query the rapidapi service using these secrets as shown below.
In order to retrieve the secrets data we are going to use the secrets context. This data can be provide as an input or environment variable to the github actions. In this workflow we are passing this data as environment variables.
[admin@fedser workflows]$ cat apisecret.yml
name: Query RAPID API Serice using secret key and token
run-name: ${{ github.actor }} is Querying RAPID API Service using secret key and token 🚀
on: [push]
jobs:
query-rapid-api-service:
runs-on: ubuntu-latest
steps:
- name: Check out repository code
uses: actions/checkout@v3
- name: Install Python
uses: actions/setup-python@v4
with:
python-version: '3.11'
- name: Install pip packages
run: pip install requests
- name: query rapidapi service
run: python lovecal.py "$api_key" "$api_token"
env:
api_key: ${{ secrets.api_key }}
api_token: ${{ secrets.api_token }}
Step5: Push Changes
Now that we are ready with the changes. Let’s push our changes to the github repository.
[admin@fedser learngit]$ git add .
[admin@fedser learngit]$ git commit -m "api query using secret key and token"
[admin@fedser workflows]$ git push -u origin main
Step6: Validate Workflow
Here as you can see in our step “query rapidapi service” the python script is executed with the secret data as input arguments and provides us with the resultant output from the API service in JSON format.
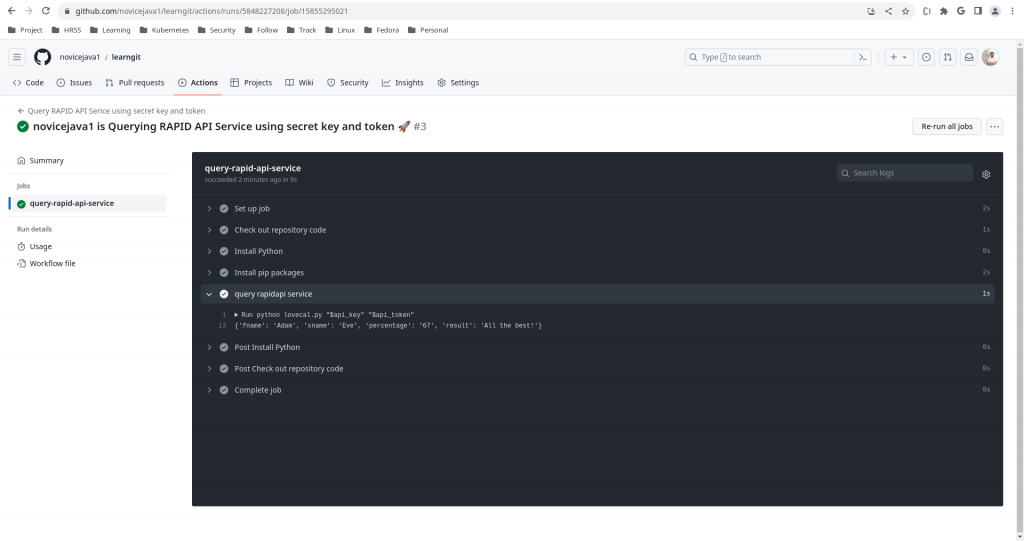
Hope you enjoyed reading this article. Thank you..
Leave a Reply
You must be logged in to post a comment.