How to Dynamically load Godot Scenes using Slider Example
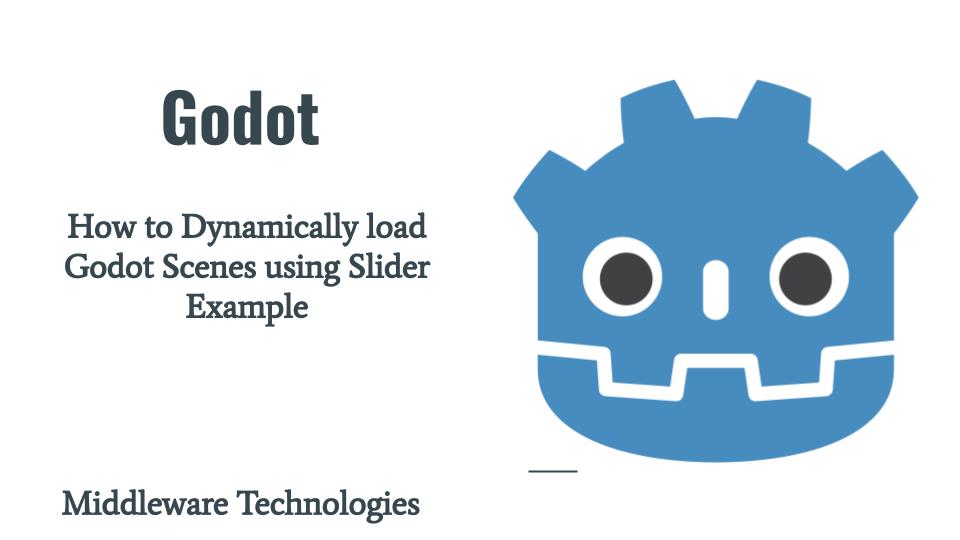
Here in this article we will demonstrate the usage of Godot Scenes and see how we can change the scene based on a condition. Also we will utilize the feature of global singleton variable to capture the input for the game using the Auto loaded variables. We will using a basic Slider game example in which we will be sliding the slabs either in left, right or top and bottom direction by initializing the respective scene on the basis on direction provided.
Test Environment
Fedora 35 workstation
Godot 3.5
If you are interested in watching the video. Here is the YouTube video on the same step by step procedure outlined below.
Procedure
Step1: Create a new Project named SliderExample
As a first step let’s create a ‘SliderExample’ project in Godot as shown below.
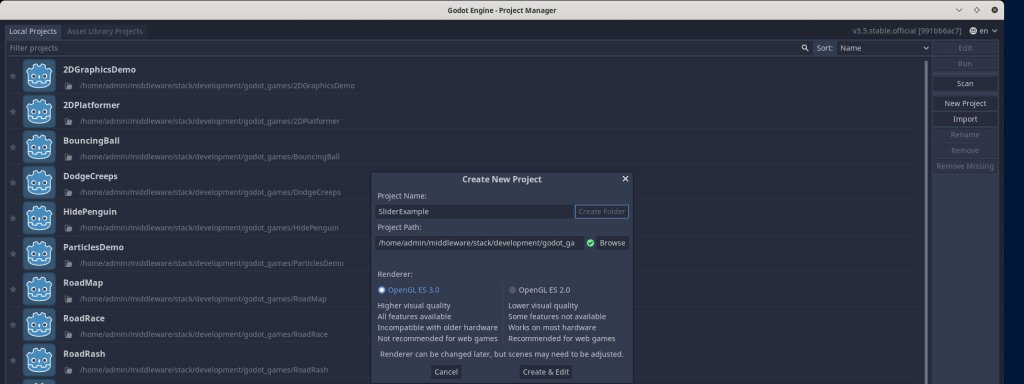
Step2: Create a MainScene
In this step we will create a MainScene for our game which will incorporate other Child Scene. We will use the ‘Node’ Object for our MainScene and rename it as ‘MainScene’. Once the MainScene node is created, let’s save the project and make the MainScene.tscn as our default scene to load.

Step3: Create a Slider Scene
In this step we will create a new scene named ‘Slider’ using ‘Node2D’ object with a ‘Sprite’ and ‘CollisionShape2D’ node objects as its child. We will add a texture with the following ‘slider.png’ image to the Sprite node object and provide a collision shape for collision detection for the sprite and save the scene. Here is the screenshot below of the scene.
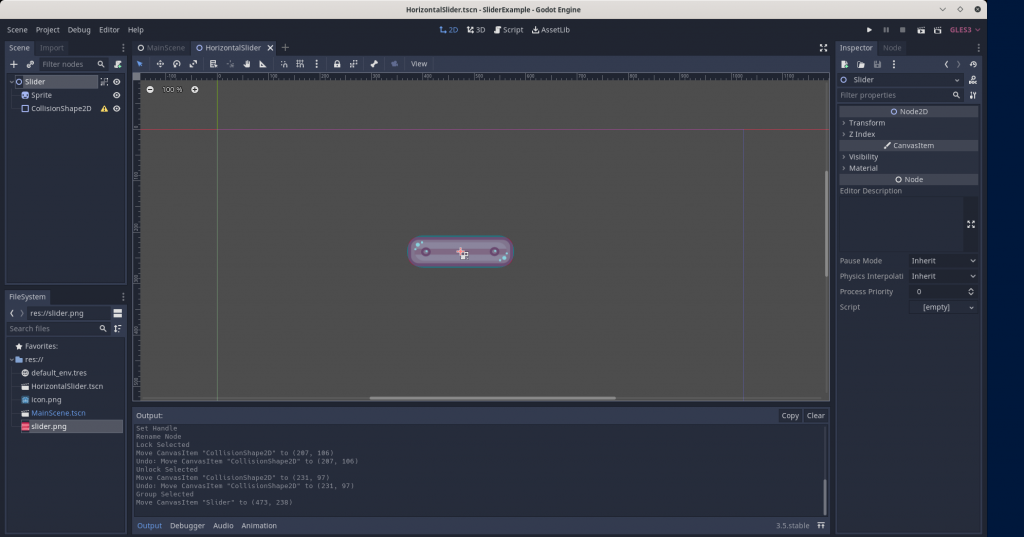
Step4: Create a Horizontal Slider Scene
Here we are going to create a new scene named ‘HorizontalSlider’ and Instantiate three instances of Slider as Child nodes of the Horizontal Slider as shown below.
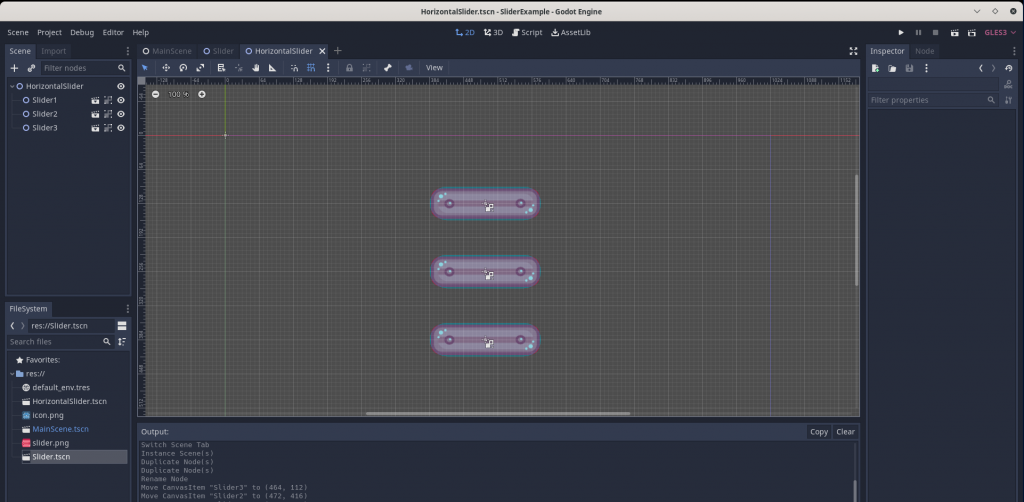
Step5: Create a Vertical Slider Scene
Similar to Step4, Here we are going to create a new scene named ‘VerticalSlicer’ and Instantiate three instances of Slider as Child nodes of the Vertical Slider as shown below.
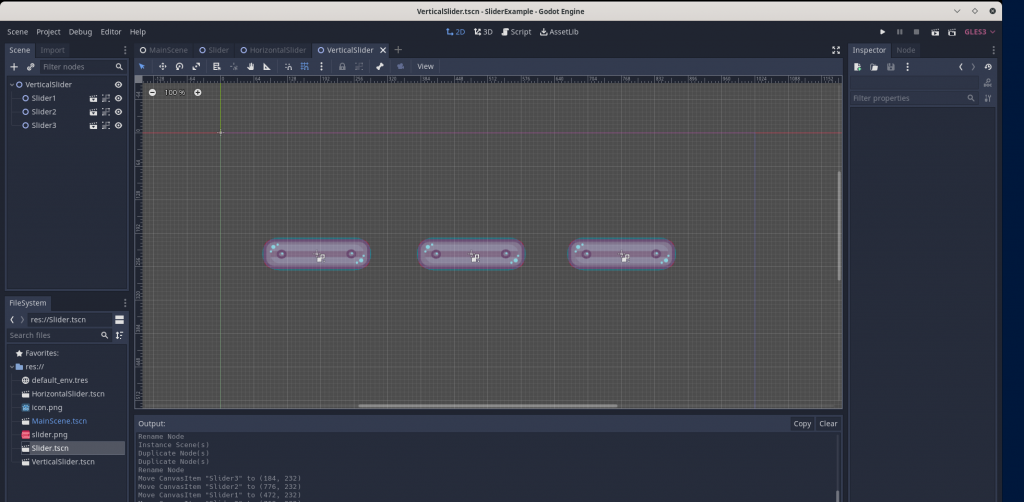
Step6: Attach GDScript for the MainScene
Here in this MainScene.gd script we are loading the appropriate Scene based on the direction variable that we are setting. If the direction is either “UP” or “DOWN” then “VerticalSlider.tscn” scene will be loaded, otherwise “HorizontalSlider.tscn” will be loaded.
Also we will loading this MainScene under Project – Project Settings – AutoLoad as shown below to make the direction variable globally available for the other scenes.
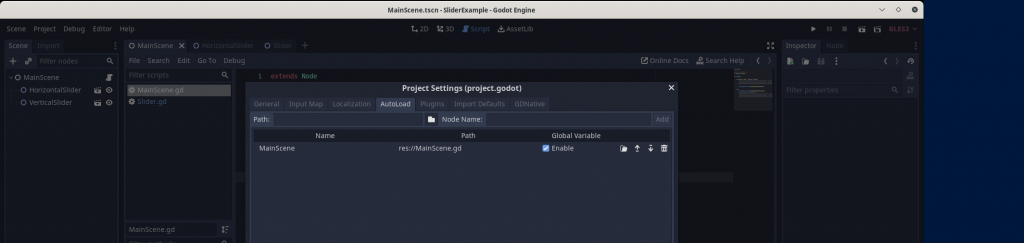
MainScene.gd
extends Node
#export var direction = "UP"
var direction = "RIGHT"
func _ready():
if direction == "UP" or direction == "DOWN":
get_tree().change_scene("res://VerticalSlider.tscn")
else:
get_tree().change_scene("res://HorizontalSlider.tscn")
Step7: Attach GDScript for the Slider Scene
Here is the Slider.gd script which based on the global singleton variable ‘direction’ moves the slides either in left, right, top or bottom direction. This global singleton variable ‘direction’ is initialized in our MainScene.gd script as shown in above step.
Slider.gd
extends Node2D
var direction = MainScene.direction
var speed = 400
var screen_size
var nodes
func _process(delta):
print("direction")
print(direction)
screen_size = get_viewport_rect().size
print(screen_size)
print(rotation)
if direction == "UP":
var velocity = Vector2.UP * speed
move(direction, velocity, delta)
elif direction == "RIGHT":
var velocity = Vector2.RIGHT * speed
move(direction, velocity, delta)
elif direction == "LEFT":
var velocity = Vector2.LEFT * speed
move(direction, velocity, delta)
else:
var velocity = Vector2.DOWN * speed
move(direction, velocity, delta)
func move(direction, velocity, delta):
#pass
print(velocity)
print(position)
position = position + velocity * delta
print(position)
position.x = clamp(position.x, 0, screen_size.x)
position.y = clamp(position.y, 0, screen_size.y)
if direction == "UP":
if position.y == 0:
position.y = screen_size.y
elif direction == "RIGHT":
if position.x == screen_size.x:
position.x = 0
elif direction == "LEFT":
if position.x == 0:
position.x = screen_size.x
else:
if position.y == screen_size.y:
position.y = 0
Step8: Run the Game
Now that you game is ready. you can launch the game using the Play Project. Make sure you have set the MainScene to be the default scene to launch on startup. You should now be able to see the slabs moving the direction mentioned and the respective scene getting instantiated.
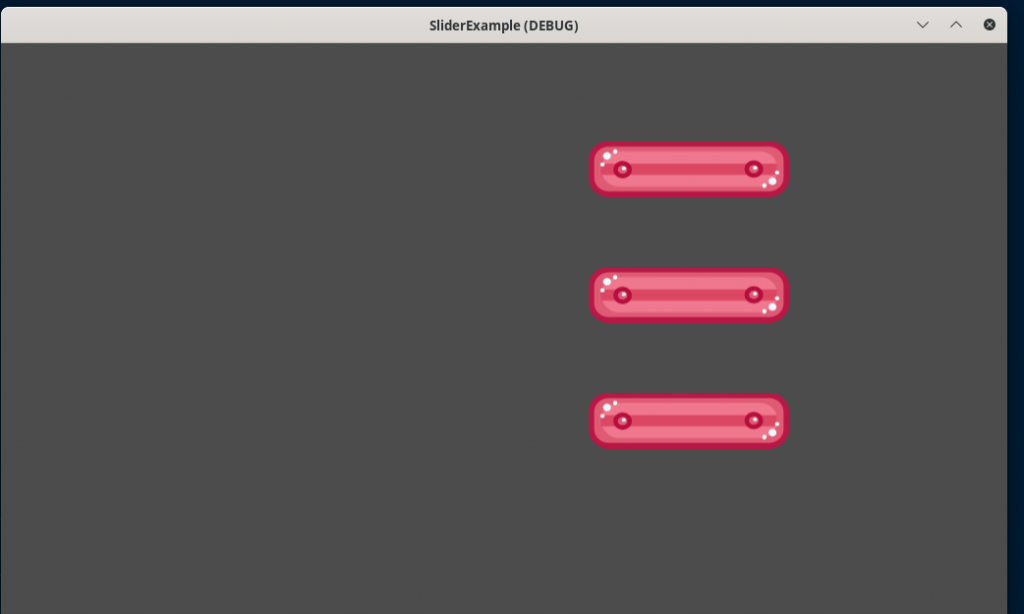
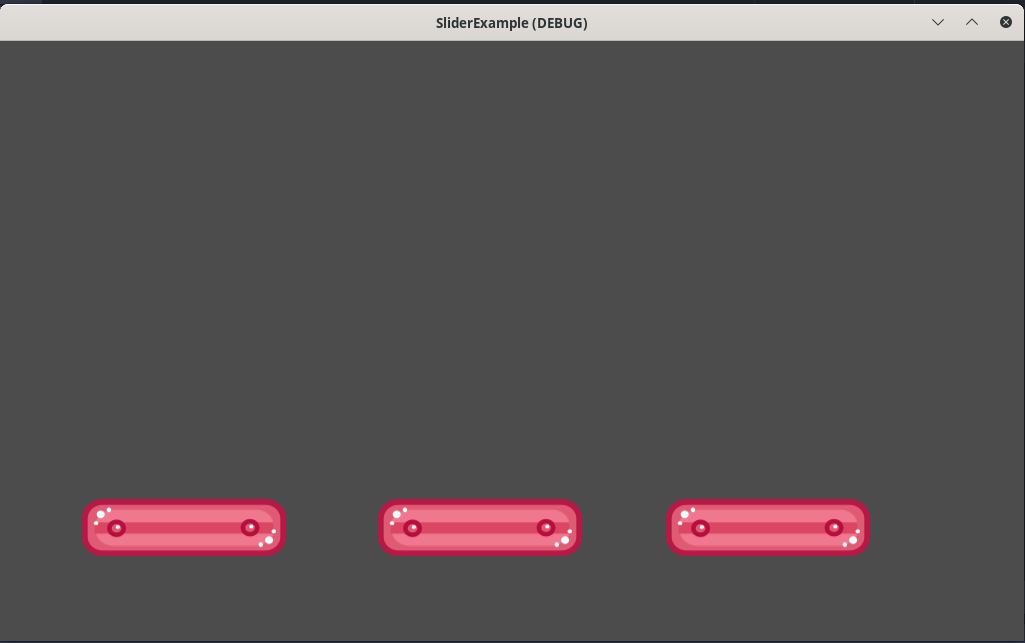
Hope you enjoyed reading this article. Thank you..
Leave a Reply
You must be logged in to post a comment.