What are the Python Object Types
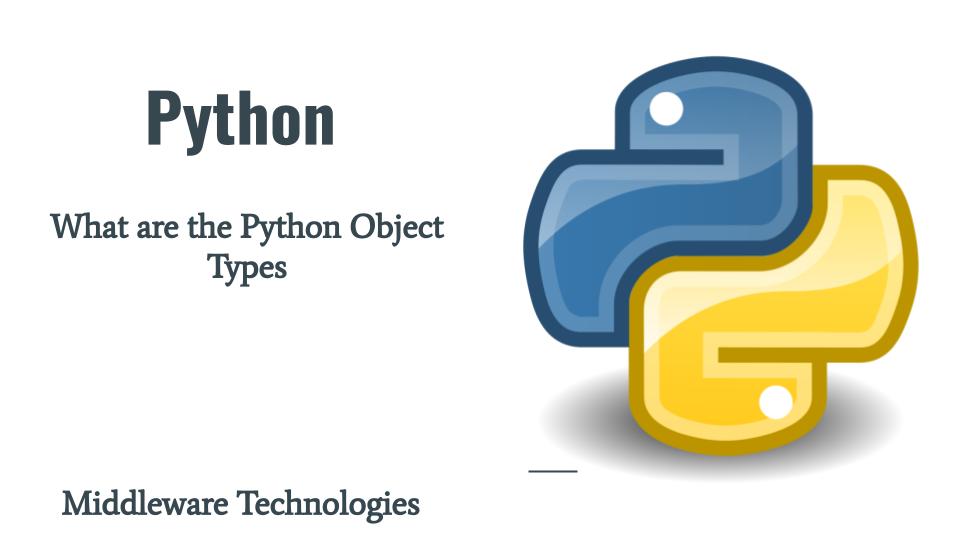
In Python the data we interact with is in the form of objects and these data objects have associated operations. We manipulate the data objects using the associated operations. In basic terms we do things with stuff in Python where the stuff is data and the things that we can carry out with the data is the associated operations. The objects can be built-in objects or custom objects built using class and extension libraries. In short everything is an object in Python.
So What does Python Program Consist Of
- Informally its a .py extension file also called module file in more formal terms
- Module files contain statements
- Statements contain expressions which results in an output
- Expressions consist of objects and they create, manipulate or process objects
Built-in Object Types
Numbers
Numbers are the most basic object types that we deal with literally in any programming language. We can have integers, floating point numbers, complex numbers, fixed precision decimals and rationals to name a few. They are used to represent most of the numeric quantities.
Here are few examples showing the type of the object for a numeric literal and some basic operations that we can carry out with numbers.
>>> type(8)
<class 'int'>
>>> 8 * 8 * 8
512
>>> 8 ** 3
512
Let’s use the built-in math module which provides us with some useful functions as shown below.
>>> import math
>>> dir(math)
['__doc__', '__file__', '__loader__', '__name__', '__package__', '__spec__', 'acos', 'acosh', 'asin', 'asinh', 'atan', 'atan2', 'atanh', 'ceil', 'comb', 'copysign', 'cos', 'cosh', 'degrees', 'dist', 'e', 'erf', 'erfc', 'exp', 'expm1', 'fabs', 'factorial', 'floor', 'fmod', 'frexp', 'fsum', 'gamma', 'gcd', 'hypot', 'inf', 'isclose', 'isfinite', 'isinf', 'isnan', 'isqrt', 'lcm', 'ldexp', 'lgamma', 'log', 'log10', 'log1p', 'log2', 'modf', 'nan', 'nextafter', 'perm', 'pi', 'pow', 'prod', 'radians', 'remainder', 'sin', 'sinh', 'sqrt', 'tan', 'tanh', 'tau', 'trunc', 'ulp']
>>> math.sqrt(100)
10.0
>>> math.pi
3.141592653589793
Also we can use the built-in random module to generate a random number for usage as shown below.
>>> import random
>>> dir(random)
['BPF', 'LOG4', 'NV_MAGICCONST', 'RECIP_BPF', 'Random', 'SG_MAGICCONST', 'SystemRandom', 'TWOPI', '_ONE', '_Sequence', '_Set', '__all__', '__builtins__', '__cached__', '__doc__', '__file__', '__loader__', '__name__', '__package__', '__spec__', '_accumulate', '_acos', '_bisect', '_ceil', '_cos', '_e', '_exp', '_floor', '_index', '_inst', '_isfinite', '_log', '_os', '_pi', '_random', '_repeat', '_sha512', '_sin', '_sqrt', '_test', '_test_generator', '_urandom', '_warn', 'betavariate', 'choice', 'choices', 'expovariate', 'gammavariate', 'gauss', 'getrandbits', 'getstate', 'lognormvariate', 'normalvariate', 'paretovariate', 'randbytes', 'randint', 'random', 'randrange', 'sample', 'seed', 'setstate', 'shuffle', 'triangular', 'uniform', 'vonmisesvariate', 'weibullvariate']
>>> random.randint(0, 10) # Generating a random number between 0 to 10
6
Strings
Strings are another most basic object types which we use mostly capturing the textual information. For a system admin it may be the servers name which he wants to capture and for a music lover it can be a collection data related a particular music track. All these information can be captured using the strings object type. Strings are a kind of sequence which consist of an ordered collection of objects.
Let’s try to represent some simple strings and generic sequence operations that can be applied on it.
>>> movie = "Harry Potter"
>>> type(movie)
<class 'str'>
>>> dir(movie)
['__add__', '__class__', '__contains__', '__delattr__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__getnewargs__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__iter__', '__le__', '__len__', '__lt__', '__mod__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__rmod__', '__rmul__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', 'capitalize', 'casefold', 'center', 'count', 'encode', 'endswith', 'expandtabs', 'find', 'format', 'format_map', 'index', 'isalnum', 'isalpha', 'isascii', 'isdecimal', 'isdigit', 'isidentifier', 'islower', 'isnumeric', 'isprintable', 'isspace', 'istitle', 'isupper', 'join', 'ljust', 'lower', 'lstrip', 'maketrans', 'partition', 'removeprefix', 'removesuffix', 'replace', 'rfind', 'rindex', 'rjust', 'rpartition', 'rsplit', 'rstrip', 'split', 'splitlines', 'startswith', 'strip', 'swapcase', 'title', 'translate', 'upper', 'zfill']
>>> movie[5:]
' Potter'
>>> movie[0:5]
'Harry'
>>> movie[:]
'Harry Potter'
Strings which are a sequence type support all the basic operations which can be applied on sequences, that are slicing, indexing, concatenation, repetition. Every object in python is classified as either immutable or mutable. Numbers, Strings and Tuples which are the core types are immutable objects. In addition to the generic sequence operations, strings also have type specific operations also called methods of their own. Here are the few examples below.
>>> movie.upper()
'HARRY POTTER'
>>> movie.lower()
'harry potter'
>>> movie.split(" ")
['Harry', 'Potter']
>>> movie.isascii()
True
>>> movie.find("tt")
8
>>> movie.find("y")
4
Lists
Lists are positionally ordered collections of arbitrary typed objects. They are mutable and they don’t have any fixed size. List are also a type of sequences so they support all the sequence operations. Here is an example list of servers which we will take as an example and apply some sequence operations on it.
>>> servers = ["fedser34", "fedser35", "fedser36"]
>>> dir(servers)
['__add__', '__class__', '__class_getitem__', '__contains__', '__delattr__', '__delitem__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__gt__', '__hash__', '__iadd__', '__imul__', '__init__', '__init_subclass__', '__iter__', '__le__', '__len__', '__lt__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__reversed__', '__rmul__', '__setattr__', '__setitem__', '__sizeof__', '__str__', '__subclasshook__', 'append', 'clear', 'copy', 'count', 'extend', 'index', 'insert', 'pop', 'remove', 'reverse', 'sort']
>>> len(servers)
3
>>> servers[0:2]
['fedser34', 'fedser35']
Also as we discussed in the Strings section, Lists also contain their own Type Specific operations called methods. Lets apply some of these methods on the list and see the results.
>>> servers.pop(1)
'fedser35'
>>> servers
['fedser34', 'fedser36']
>>> servers.extend(["fedser35"])
>>> servers
['fedser34', 'fedser36', 'fedser35']
>>> servers.sort()
>>> servers
['fedser34', 'fedser35', 'fedser36']
Lists can contain nested lists as their items and also they support list comprehension expression which is a powerful tool to process structures like the matrix.
Dictionaries
Python Dictionaries are also know as mappings. They are a collection objects but they are stored and referenced using key instead of a relative position or index. Python Dictionaries are also mutable like list as they can be changed in place and can grow and shrink on demand. Mappings are not positionally ordered like the lists or strings.
Let’s create a simple dictionary of a student capturing his details as shown below.
>>> student1 = {'firstname': 'Alice', 'lastname': 'Wonderland', 'age': 23}
>>> type(student1)
<class 'dict'>
>>> student1['age'] # Fetch the age of the student
23
>>> student1['class'] = 'Graduate' # Extend the dictionary by adding a new key value
>>> print(student1)
{'firstname': 'Alice', 'lastname': 'Wonderland', 'age': 23, 'class': 'Graduate'}
Tuples
Tuples are like list but they cannot be changed. They are immutable object types. Tuples are used to captured a fixed collection of items like the months, days etc.
Here are some examples of Tuples.
>>> days = ("Monday", "Tuesday", "Wednesday", "Thursday", "Friday", "Saturday", "Sunday")
>>> type(days)
<class 'tuple'>
>>> dir(days)
['__add__', '__class__', '__class_getitem__', '__contains__', '__delattr__', '__dir__', '__doc__', '__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__getnewargs__', '__gt__', '__hash__', '__init__', '__init_subclass__', '__iter__', '__le__', '__len__', '__lt__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__', '__rmul__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__', 'count', 'index']
As tuples are also a kind of sequences all the normal sequence related operation can also be applied to tuples like indexing, slicing.
>>> days[0:5]
('Monday', 'Tuesday', 'Wednesday', 'Thursday', 'Friday')
>>> len(days)
7
Tuples also have type specific methods but only a few as shown below.
>>> days.index("Tuesday")
1
>>> days.count("Friday")
1
These are some of the core built-in data types that we can apply and use in python application programming.
Hope you enjoyed reading this article. Thank you..
Leave a Reply
You must be logged in to post a comment.