Getting Started with Python
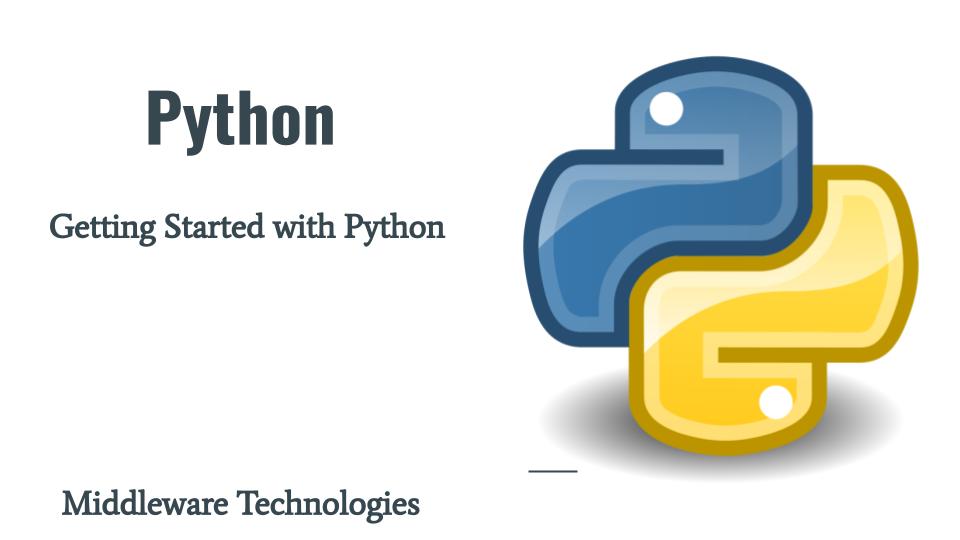
As per the StackOverflow 2022 most popular technologies survey Python is still in the Top 5 list of popular languages and also 3rd most popular language for learning to code. Python in short is a general purpose programming language with procedural, functional, and object-oriented paradigms. Its an Open Source system developed and maintained by Python Software Foundation.
Why is Python Still Popular
- Indentation of code is one of the most important thing that makes Python so popular. The code written with proper indentation make it easy to read and maintain. Also it makes the code reusable for other developer with ease. Python also has support for Object Oriented and function programming which helps in software re usability.
- Software or Programs written in Python are much smaller and concise compared to the programs written in other languages like C or C++. Also it abstracts the compilation step which is required to be executed in some statically typed languages and help boost the productivity in software development.
- Python programs are portable and run without any change on all major computer platforms.
- Python comes with a large collection of standard libraries in built. These built in libraries help in interacting with the Operating system platform and networking system by abstracting away the low level details of the system. The built in libraries also provide the programmer with some of the core libraries for interactivity with the filesystem, working with mathematical problem etc.
- Python is also popular for system integration. It can be used to call the native code written in C or C++ for some of the time critical tasks. Also it can be called from C, C++ or Java code to name a few.
Python’s Downside or Drawback
The only downside or drawback that we can think about is its execution speed. Though in today era with unlimited system resources like CPU and Memory this is rarely a problem but in some specific domains like mathematics or scientific domain where execution speed is critical the critical tasks can be offloaded to be executed in native programming languages for optimized execution speed.
And Also, rapid evolution and changes in Python and release of new Python version makes it difficult to keep up with the new features and changes.
Python Applications
System Programming
Python is used for system programming in which it uses the built-in interfaces to the OS. The built-in libraries help in building administrative tools and utilities for interacting with the system low level architecture, for file management, for file parsing, compression, socket and network management to name a few.
GUI Programming
Python is also popular for GUI program application. Kivy, Tkinter and PyQt are some of the popular python frameworks that help in developing GUI based applications.
Web Programming
Python comes with built-in libraries for working on various networking tasks like urllib2. Some of the popular web frameworks that help in web development or Django, Bottle, Flask to name a few.
Component Integration
Python’s code can be extended by and embedded in C and C++ systems for optimizing the execution speed and for integrating disparate systems written in heterogeneous languages. Some of the tools like SWIG and SIP code generators automate the work needed to link compiled components into Python for use in scripts.
Database Programming
Python provides with interfaces for some of the popular relational database systems like MySQL, Oracle, PostgreSQL to name a few.
Numeric and Scientific Programming
NumPy, SciPy and Pandas are some of the popular python extensions which support in the developing application in the Scientific and Mathematics Domain.
Game Programming
Popular Python frameworks like Pygame and Panda3D can be used for Game development.
Python is so extensive with built in libraries and the external third party libraries that it almost used and application in any domain.
Python Technical Strengths
- Python support procedural, functional and object oriented programming paradigms. Python programs can subclass classes implemented in C++, Java and C#. It class models support polymorphism, operator overloading, and multiple inheritance. Its functional programming features include generators, comprehensions, closures, maps, decorators and lambda functions etc.
- Python is Open source system free to use and distribute.
- Python is portable. Its available on most of the popular Operation system platforms. Python programs using the core language and standard libraries run the same on Linux, Windows, and most other systems with a Python interpreter.
- Python provides with all the tools that are required for scripting along with more advanced software engineering tools found in compiled languages.
- Python is easy to program, easy to use and easy to learn.
Python Installation
Python is by default installed on almost all the popular linux platform. Here i am working on a Fedora workstation and its installed by default. We can verify its installation as shown below.
[admin@fedser ~]$ which python
/usr/bin/python
Python installation installs the Python Interpreter program along with core built-in libraries. We can launch the Python interpreter to run our programs interactively as shown below.
[admin@fedser ~]$ python
Python 3.10.5 (main, Jun 9 2022, 00:00:00) [GCC 11.3.1 20220421 (Red Hat 11.3.1-2)] on linux
Type "help", "copyright", "credits" or "license" for more information.
>>>
Now we ready to execute our code at the Python Interactive terminal as shown below. Let’s try to print a pattern as shown below. Make sure you keep the indentation for the code block as shown below.
>>> for i in range(10):
... for j in range(i):
... print("*", end="")
... print()
...
*
**
***
****
*****
******
*******
********
*********
Python Interactive terminal is great for beginner to start learning python and also to test and debug your code snippets. To exit from the interactive shell prompt, just press Ctrl + D and you get to your original Linux shell prompt.
Hope you enjoyed reading this article. Thank you..
Leave a Reply
You must be logged in to post a comment.