Learning Python Classes and OOP’s programming concepts
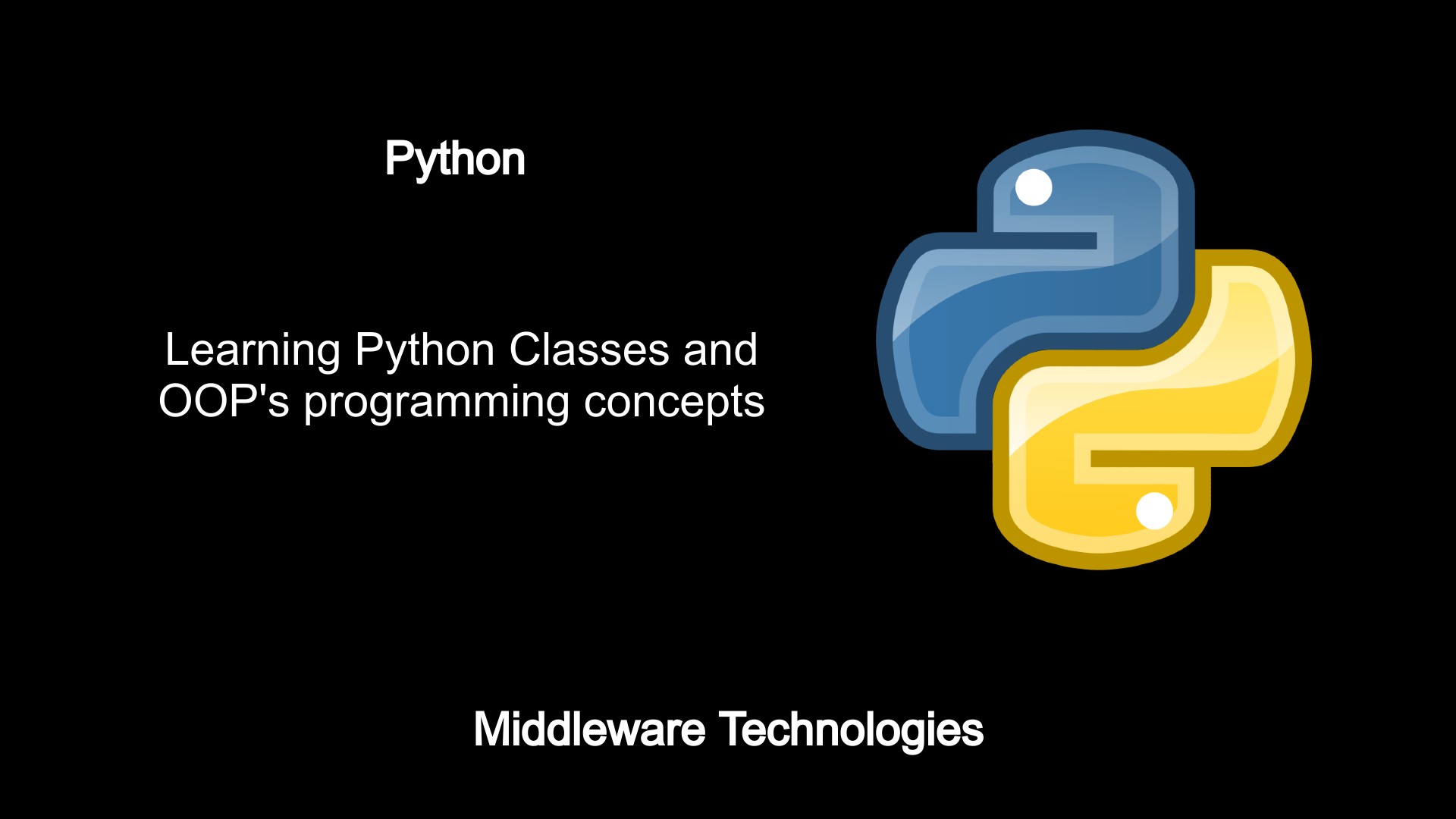
Test Environment
Fedora 31 installed
Python 3.7 and later
What are Python Classes
Python Classes are an advanced programming concepts which provide OOP’s features. Classes package program logic and program data into a single unit. They define a new namespace like functions and modules. The main difference between Python Classes and Modules/functions is that Modules objects instantiated and loaded into memory once. In case any changes done to the module state (ie. attributes) we need to reload the module for the changes to take effect.
But Python Classes are used to instantiate multiple instances of the same class with different object state. They also allow for customization through inheritance which is not possible with Modules or functions.
Here in this article we will look into the below tasks.
- How to build a basic python class
- How to instantiate multiple instances of a python class
- How to incorporate inheritance into python class
- How to Customize class function definition by overriding its base class function definition
Procedure
Step1: Build a basic python class
Here is our basic class greet. This class definition is not much of use but let see some Python Class related details with it. The first statement ‘class greet:’ when its executed it first creates a class object greet then it proceeds to execute the print statement. Once the Class definition is executed we proceeding with our main section execution with a print statement.
The statement ‘g1 = greet()’ is actually instantiating a greet class object. So, as of now we have two types of object one is class object and the other one is the instance object which we can check with type statement shown below.
$ cat simpleoop.py
#!/usr/bin/env python
class greet:
print("Hello Greeting!!")
print("Executing the main section")
g1 = greet()
print(type(greet))
print(type(g1))
$ ./simpleoop.py
Hello Greeting!!
Executing the main section
"class 'type'"
"class '__main__.greet'"
Step2: Instantiate multiple instances of a python class
In the above step we have seen what a basic class looks like and what are the different types of objects that get created when a Class definition is executed and Class is instantiated. Now, lets see how we can instantiate two different classes with different state of their own.
Here in the below class definition we have modified and included a function definition ‘getMessage’ which basically return a message. The important thing to note here is the first argument ie. self. Its actually referring to instance object from where this function is called. By default this is the first argument in every function definition within the class.
Now that we have our function ready which accepts the instance object ie ‘self’ parameter by default and our custom ‘msg’ parameter. Lets instantiate two different objects ‘g1’ and ‘g2’ as shown below and call the getMessage() function definition with a msg argument.
As you can see from the below statement function definition are called using the . notation where in the left side of the . is instance object and right side is the function name (ie. object.attribute where our attribute is a method now). This statement is same as class.method(object). Its basically state we are calling a function with first argument by default an instance object.
Lets execute and see the two different message that we have passed as output of the print statement results.
$ cat simpleoop.py
#!/usr/bin/env python
class greet:
#print("Hello Greeting!!")
def getMessage(self, msg):
return "Hello : " + msg
print("Executing the main section")
g1 = greet()
g2 = greet()
#print(type(greet))
#print(type(g1))
print(g1.getMessage("Good Morning"))
print(g2.getMessage("Good Afternoon"))
$ ./simpleoop.py
Executing the main section
Hello : Good Morning
Hello : Good Afternoon
Step3: Incorporate inheritance into Python Class
Inheritance is the most import aspect of Python Classes. Python comes with a lot of library included. These libraries can bey Modules with various functions and class definitions. There are a lot of libraries or framework already developed and are ready to be used or extended in our program. Example Python Django framework comes with pre-built libraries and classes for web application development, Python Kivy framework is used for Multi touch GUI application which has a lot of Modules already developed which can be extended further in our code to meet the specific requirement.
There are a lot of tools and frameworks already developed, we can just inherit them and extend their function to customize the behaviour as per our requirement.
Let’s create a new class student which will accept the name and greet them with a message. Here we are extending the student class with greet class with statement ‘class student(greet):’. student is now a subclass of greet which is a parent class. Also note, we have modified our getMessage() function definition to return a standard welcome message.
In student class we are basically inheriting the greet class and all its attributes. As we have extended our student class, now we are able to call the getMessage function which is actually available in greet class but now also available or accessible from within the student class.
object.attribute searches a tree of linked objects looking for the first appearance of attribute that it can find. Its first looks in the leaf subclass then all classes above it, bottom to top and left to right if there are multiple top level parent classes for one class.
Lets execute and see the different greet messages output with student name in it.
$ cat simpleoop.py
#!/usr/bin/env python
class greet:
#print("Hello Greeting!!")
def getMessage(self):
return "Welcome to Python Class"
class student(greet):
def greetStudent(self, name):
return "Hello : " + name + " " + self.getMessage()
print("Executing the main section")
s1 = student()
s2 = student()
print(s1.greetStudent("Laura"))
print(s2.greetStudent("William"))
$ ./simpleoop.py
Executing the main section
Hello : Laura Welcome to Python Class
Hello : William Welcome to Python Class
Step4: Customize class function definition by overriding its base class function definition
We have seen inheritance in previous step, Let’s now override the parent class greet ‘getMessage()’ function in our subclass ‘student’. As you can see from below we have customized the getMessage() function in student class by overriding it from base class greet. Here in this overridden function we have modified the Welcome message.
Lets execute and see the customized greet message output with student name in it.
$ cat simpleoop.py
#!/usr/bin/env python
class greet:
#print("Hello Greeting!!")
def getMessage(self):
return "Welcome to Python Class"
class student(greet):
def getMessage(self):
return "Welcome to Python Django Class"
def greetStudent(self, name):
return "Hello : " + name + " " + self.getMessage()
print("Executing the main section")
s1 = student()
s2 = student()
print(s1.greetStudent("Laura"))
print(s2.greetStudent("William"))
$ ./simpleoop.py
Executing the main section
Hello : Laura Welcome to Python Django Class
Hello : William Welcome to Python Django Class
Hope you enjoyed this article. Thank you..
Leave a Reply
You must be logged in to post a comment.