How to develop a simple Python Kivy bounce ball application
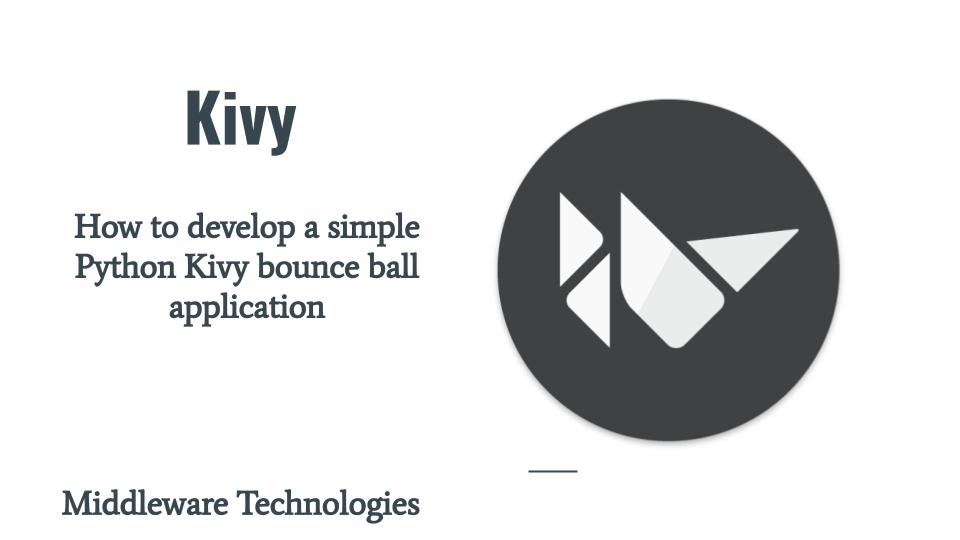
Here in this article we will try to build a very basic Kivy bounce ball application.
Test Environment
Fedora 32
Python3
Kivy 1.11.1
What is Kivy
Kivy is a python framework for GUI based Application development. It is used to develop multitouch application interfaces which are compatible on various devices. Kivy uses a special language called .kv which is used to define the presentation logic and to separate it from the Python which can used for writing business logic and dynamic content generation for interfaces.
Here is the complete source code for this simple game available on github.
Procedure
Step1: main.py which extends the App base class and other Widget class to define the business logic
[admin@feddesk brick]$ cat main.py
#!/usr/bin/env python
from kivy.app import App
from kivy.uix.widget import Widget
from kivy.properties import NumericProperty, ReferenceListProperty, ObjectProperty
from kivy.vector import Vector
from kivy.clock import Clock
from random import randint
class PongPaddle(Widget):
score = NumericProperty(0)
pass
class PongBall(Widget):
velocity_x = NumericProperty(0)
velocity_y = NumericProperty(0)
velocity = ReferenceListProperty(velocity_x, velocity_y)
def move(self):
self.pos = Vector(*self.velocity) + self.pos
class PongGame(Widget):
ball = ObjectProperty()
player = ObjectProperty()
#score = NumericProperty()
def serve_ball(self):
self.ball.center = self.center
self.ball.velocity = Vector(4, 0).rotate(randint(0, 360))
def update(self, dt):
self.ball.move()
if (self.ball.y < self.player.top) or (self.ball.top > self.height):
self.ball.velocity_y *= -1
if (self.ball.x < 0) or (self.ball.right > self.width):
self.ball.velocity_x *= -1
if self.ball.y < self.player.top:
if self.player.x < self.ball.x < self.player.right:
#print(self.player.score)
self.player.score += 1
#self.serve_ball()
def on_touch_move(self, touch):
if touch.x < 50:
self.player.x = touch.x
if touch.x > 50:
self.player.right = touch.x
class PongApp(App):
def build(self):
game = PongGame()
game.serve_ball()
Clock.schedule_interval(game.update, 1.0 / 60.0)
return game
if __name__ == "__main__":
PongApp().run()
Step2: kivy file pong.kv which defines the presentation logic for the Widgets
Main Widget - PongGame
Ball Widget - PongBall
Player Widget - PongPaddle
pong.kv
[admin@feddesk brick]$ cat pong.kv
# File name: pong.kv
:
size: 20, 20
canvas:
Ellipse:
pos: self.pos
size: self.size
:
size: 100, 10
pos: self.center_x - 50, 0
canvas:
Rectangle
pos: self.pos
size: self.size
:
ball: pong_ball
player: player1
PongPaddle:
id: player1
y: 20
center_x: root.center_x
Label:
font_size: 20
center_x: root.width - 30
top: root.top - 50
text: "Score"
Label:
font_size: 20
center_x: root.width - 30
top: root.top - 70
text: str(root.player.score)
PongBall:
id: pong_ball
pos: self.parent.center_x - 10, 10
Step3: Screenshot of the Game demo
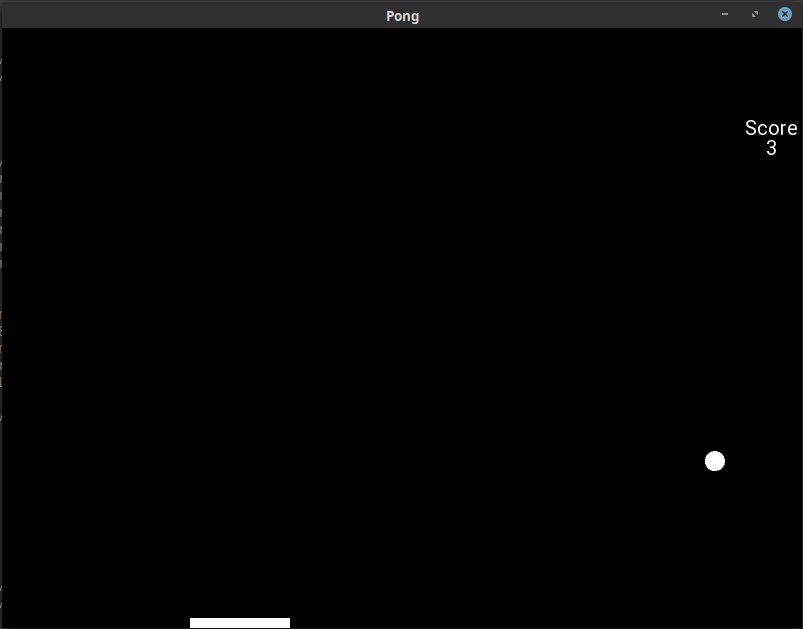
For more detailed explanation of kivy concepts please go through the Kivy documentation for Pong Game Application as a starting point.
Hope you enjoyed reading this article. Thank you..
Leave a Reply
You must be logged in to post a comment.