How to create Models in Python Django
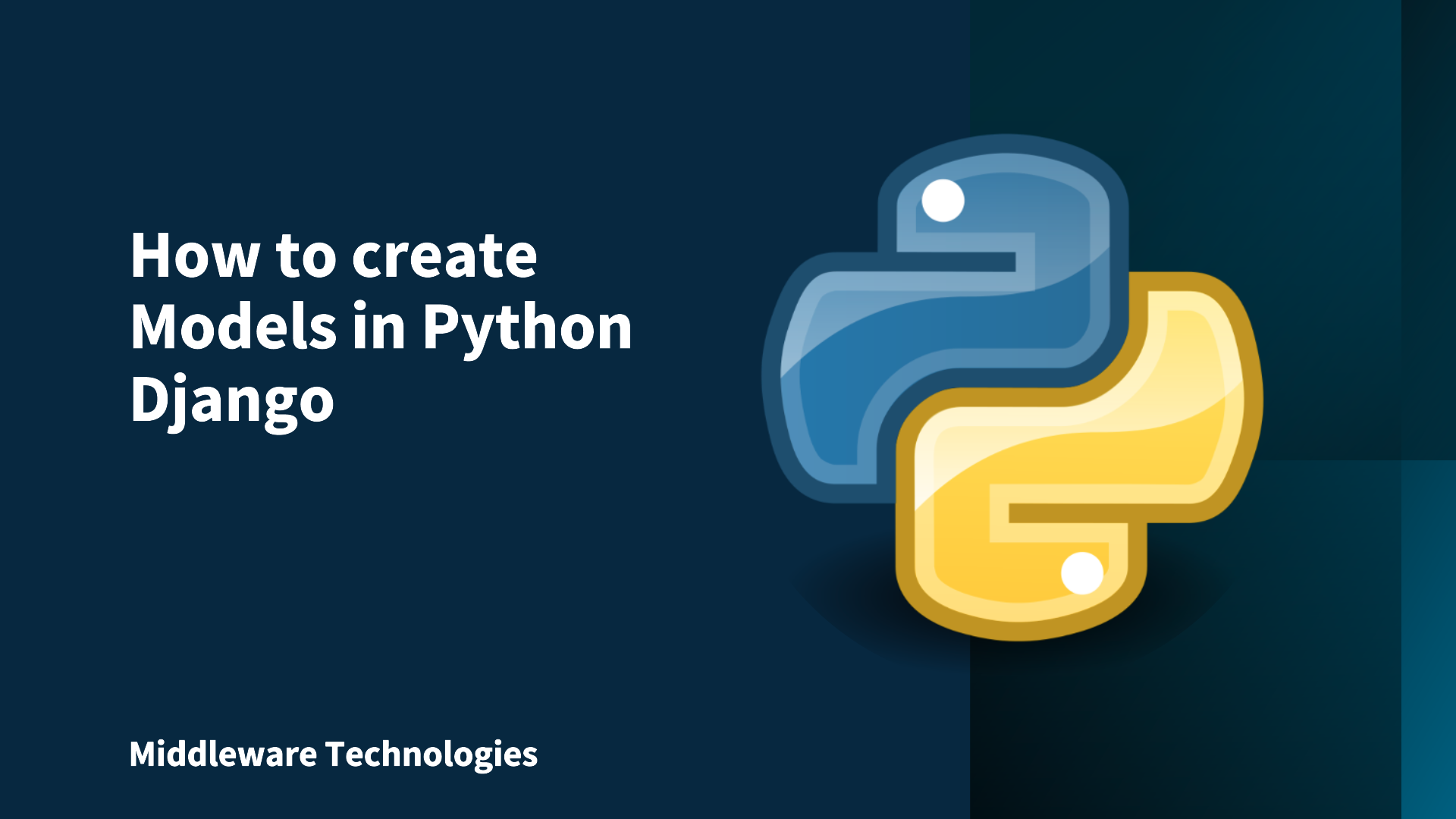
Here in this article we will try to use the Django Models as blueprints for creating the Database tables using the Django utilities.
Test Environment
Ubuntu 18.04
Python 3.5 and later
pip3 latest version
Django 2.1 and later
We will see how we can use the Django Models to layout the Models Objects as tables in the Databases. By default in the Django Projects settings.py the default SQLLite Database is configured for usage. We can either use the default Database or we can configure the setting to point to a different Database if we wish to connect to a different Database for configuration.
Procedure
Step1: Verify that the Latest version of Django is installed
user@c531f04d55ac:/projects$ python -m django --version
2.1.5
Step2: Verify that the Default SQLLite Database is configured in the settings.py
Here we have already created a project by name mysite as per the quickstart documents and went into the project folders as below where we can find the setting.py file which contains the project specfic settings.
user@c531f04d55ac:/projects/mysite/mysite$ grep -A 10 Database settings.py
# Database
# https://docs.djangoproject.com/en/2.1/ref/settings/#databases
DATABASES = {
'default': {
'ENGINE': 'django.db.backends.sqlite3',
'NAME': os.path.join(BASE_DIR, 'db.sqlite3'),
}
}
Step3: Create a new Application called Students
user@c531f04d55ac:/projects/mysite$ python manage.py startapp students
user@c531f04d55ac:/projects/mysite$ ls -ltr students/
total 20
-rw-r--r-- 1 user user 0 Apr 1 20:59 __init__.py
-rw-r--r-- 1 user user 63 Apr 1 20:59 admin.py
-rw-r--r-- 1 user user 91 Apr 1 20:59 apps.py
-rw-r--r-- 1 user user 60 Apr 1 20:59 tests.py
-rw-r--r-- 1 user user 57 Apr 1 20:59 models.py
-rw-r--r-- 1 user user 63 Apr 1 20:59 views.py
drwxr-xr-x 2 user user 25 Apr 1 20:59 migrations
Step4: Create a Students and StudentInfo models
user@c531f04d55ac:/projects/mysite/students$ cat models.py
from django.db import models
# Create your models here.
class Students(models.Model):
full_name = models.CharField(max_length = 70)
class StudentInfo(models.Model):
name = models.ForeignKey(Students, on_delete = models.CASCADE)
gender = models.CharField(max_length = 10)
standard = models.IntegerField(default = 0)
division = models.CharField(max_length = 1)
Step5: Include the Application in the Project settings
As we have created a new Application name called students, we need to include that Application in the mysite project settings.
user@c531f04d55ac:/projects/mysite/mysite$ grep -A 10 INSTALLED_APPS settings.py
INSTALLED_APPS = [
'students.apps.StudentsConfig',
'polls.apps.PollsConfig',
'django.contrib.admin',
'django.contrib.auth',
'django.contrib.contenttypes',
'django.contrib.sessions',
'django.contrib.messages',
'django.contrib.staticfiles',
...
Step6: Make Migrations of the new Models created
Now that we have created our two models, we need to tell django to we have made some changes to the models for students Application and we would like to store those changes in the migration using the below command.
user@c531f04d55ac:/projects/mysite$ python manage.py makemigrations students
Migrations for 'students':
students/migrations/0001_initial.py
- Create model StudentInfo
- Create model Students
- Add field name to studentinfo
Step7: Validate the SQL queries that will be execute to carry out the migration
Once, we created the migrations, we can validate the SQL queries that will be executed against the database to create the Database tables related to the Models that we just created above.
user@c531f04d55ac:/projects/mysite$ python manage.py sqlmigrate students 0001
BEGIN;
--
-- Create model StudentInfo
--
CREATE TABLE "students_studentinfo" ("id" integer NOT NULL PRIMARY KEY AUTOINCREMENT, "gender" varchar(10) NOT NULL, "standard" integer NOT NULL, "division" varchar(1) NO
T NULL);
--
-- Create model Students
--
CREATE TABLE "students_students" ("id" integer NOT NULL PRIMARY KEY AUTOINCREMENT, "full_name" varchar(70) NOT NULL);
--
-- Add field name to studentinfo
--
ALTER TABLE "students_studentinfo" RENAME TO "students_studentinfo__old";
CREATE TABLE "students_studentinfo" ("id" integer NOT NULL PRIMARY KEY AUTOINCREMENT, "gender" varchar(10) NOT NULL, "standard" integer NOT NULL, "division" varchar(1) NO
T NULL, "name_id" integer NOT NULL REFERENCES "students_students" ("id") DEFERRABLE INITIALLY DEFERRED);
INSERT INTO "students_studentinfo" ("name_id", "gender", "division", "standard", "id") SELECT NULL, "gender", "division", "standard", "id" FROM "students_studentinfo__old
";
DROP TABLE "students_studentinfo__old";
CREATE INDEX "students_studentinfo_name_id_474a2cbf" ON "students_studentinfo" ("name_id");
COMMIT;
Step 8: Execute the migrations to create the model tables
user@c531f04d55ac:/projects/mysite$ python manage.py migrate
Operations to perform:
Apply all migrations: admin, auth, contenttypes, polls, sessions, students
Running migrations:
Applying students.0001_initial... OK
With the above step execute we have created empty tables related to Students and StudentInfo. Now we can use the Python shell to query our Database tables using the API
Hope you enjoyed reading this article. Thank you..
Leave a Reply
You must be logged in to post a comment.