How to update the Generic JVM Arguments using wsadmin
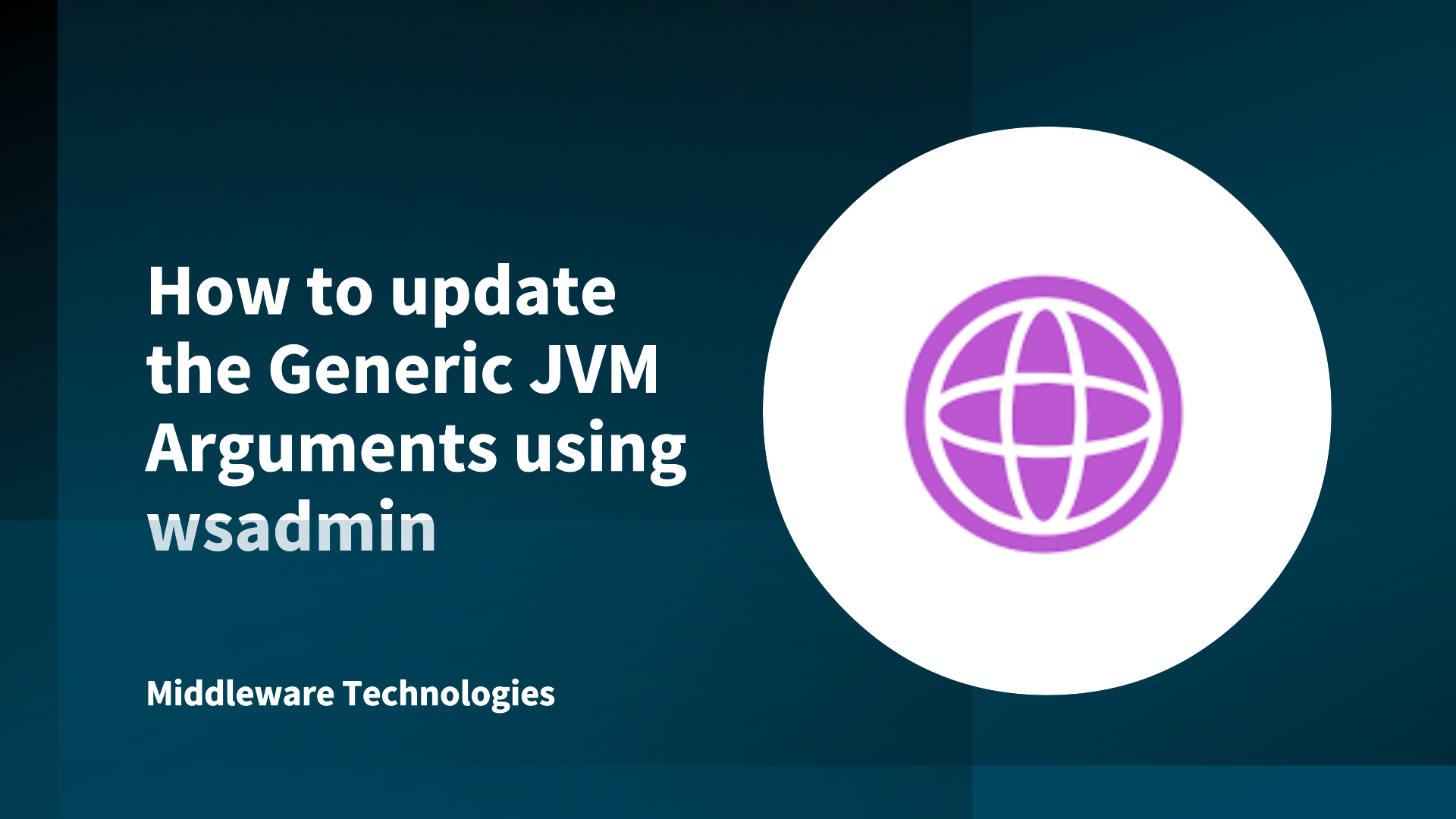
Here in this article we will try to update the Generic JVM Arguments using wsadmin.
Generic JVM arguments are the command line arguments that are passed to the JVM code that starts up the Application server process. They are used to configure and adjust how the JVM executes. Any changes to the JVM arguments requires a JVM restart for them to take affect.
Here is a sample Jython script which could be used to modify the Generic JVM arguments as per your need.
import os
import sys
import java
import java.util as util
import java.io as javaio
def usage():
print "./wsadmin.sh -lang jython -f addJVMArg_new.py <JVM_name> add <New_argument>"
print "./wsadmin.sh -lang jython -f addJVMArg_new.py <JVM_name> update"
def getJVMConfigID(jvm_name):
server_list=AdminConfig.list('Server').splitlines()
for server in server_list:
server_name=AdminConfig.showAttribute(server,'name')
if (server_name==jvm_name):
jvm_id=AdminConfig.list('JavaVirtualMachine',server)
return jvm_id
def currentJvmArguments(jvm_id):
#print "Current JVM arguments"
current_arguments=AdminConfig.showAttribute(jvm_id,"genericJvmArguments")
#print str(current_arguments)
return str(current_arguments)
def updateJvmArguments(jvm_id):
current_arguments=currentJvmArguments(jvm_id)
print str(current_arguments)
print "Input new arguments"
new_arguments=raw_input("Provide the new arguments :")
print new_arguments
print AdminConfig.modify(jvm_id,[['genericJvmArguments',new_arguments]])
#print AdminConfig.save()
def addJvmArguments(jvm_id,action,new_argument):
current_arguments=currentJvmArguments(jvm_id)
print str(current_arguments)
print "Need to add the arguments"
current_arguments=str(current_arguments) + ' ' + new_argument
print "modified arguments is as below :"
print current_arguments
print AdminConfig.modify(jvm_id,[['genericJvmArguments',current_arguments]])
#print AdminConfig.save()
if not (len(sys.argv) >= 2):
print "Usage : ",
usage()
sys.exit(1)
#######################################################################
######################## Main script ##################################
#######################################################################
jvm_name=sys.argv[0]
action=sys.argv[1]
print "JVM Name is : ", jvm_name
print "Action is : ", action
#print "new_argument is : ", new_argument
# Retrieve the JVM Config id
jvm_id=getJVMConfigID(jvm_name)
jvm_arguments=currentJvmArguments(jvm_id)
print "JVM ID is", jvm_id
print "JVM Arguments are :", jvm_arguments
if (action == "add"):
new_argument=sys.argv[2]
print "new_argument is : ", new_argument
addJvmArguments(jvm_id,action,new_argument)
else:
updateJvmArguments(jvm_id)
### END of Main Script
Output
./wsadmin.bat -lang jython -username <admin_user> -password <admin_pwd> -f addJVMArg_new.py ClusMemb_1 add "-Xnoclassgc"
WASX7209I: Connected to process "dmgr" on node XXX using SOAP connector; The type of process is: DeploymentManager
WASX7303I: The following options are passed to the scripting environment and are available as arguments that are
stored in the argv variable: "[ClusMemb_1, add, -Xnoclassgc]"
JVM Name is : ClusMemb_1
Action is : add
JVM ID is (cells/ATLADCADMIN042CellManager01/nodes/ATLADCADMIN042Node02/servers/ClusMemb_1|server.xml#JavaVirtualMachine_1432538823123)
JVM Arguments are : -Duser.region=US -Dlogging.level=DEBUG -Dlogging.level.thirdparty=DEBUG
new_argument is : -Xnoclassgc
-Duser.region=US -Dlogging.level=DEBUG -Dlogging.level.thirdparty=DEBUG
Need to add the arguments
modified arguments is as below :
-Duser.region=US -Dlogging.level=DEBUG -Dlogging.level.thirdparty=DEBUG -Xnoclassgc
./wsadmin.bat -lang jython -username <admin_user> -password <admin_pwd> -f addJVMArg_new.py ClusMemb_1 update
WASX7209I: Connected to process "dmgr" on node XXX using SOAP connector; The type of process is: DeploymentManager
WASX7303I: The following options are passed to the scripting environment and are available as arguments that are
stored in the argv variable: "[ClusMemb_1, update]"
JVM Name is : ClusMemb_1
Action is : update
JVM ID is (cells/ATLADCADMIN042CellManager01/nodes/ATLADCADMIN042Node02/servers/ClusMemb_1|server.xml#JavaVirtualMachine_1432538823123)
JVM Arguments are : -Duser.region=US -Dlogging.level=DEBUG -Dlogging.level.third party=DEBUG -Dxxx=yyy -Xnoclassgc
-Duser.region=US -Dlogging.level=DEBUG -Dlogging.level.thirdparty=DEBUG -Dxxx=yyy -Xnoclassgc
Input new arguments
-Duser.region=US -Dlogging.level=ERROR -Dlogging.level.thirdparty=DEBUG -Xnoclassgc
Hope you enjoyed reading this article. Thank you..
Leave a Reply
You must be logged in to post a comment.