How to use Jakarta Context and Dependency Injection feature
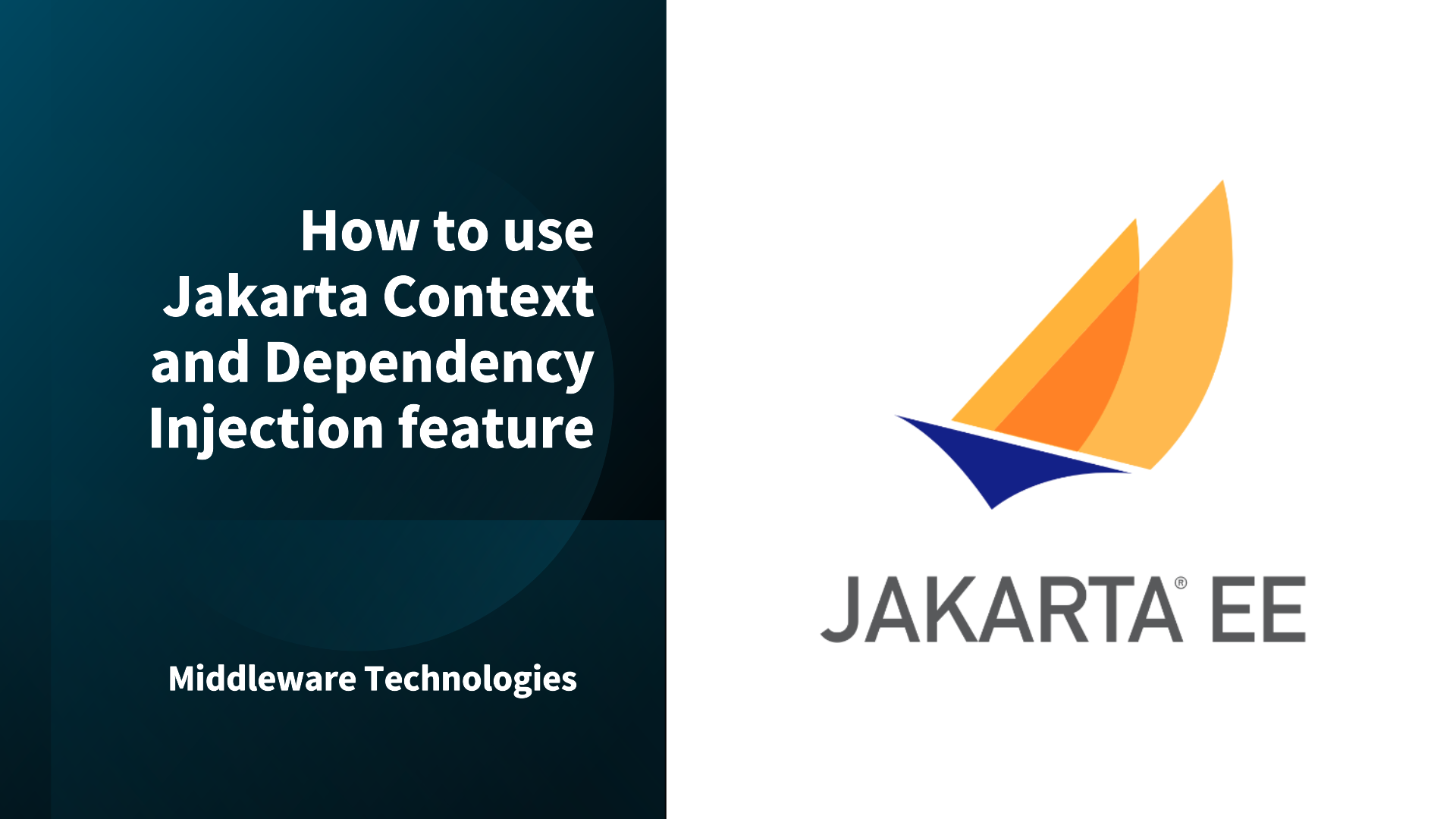
Here in this article we will try to use Jakarta CDI feature to convert a java class to a managed bean and see how it can be injected into dependent java objects.
Test Environment
- OpenJDK 17.0.13
- Eclipse GlassFish 7.0.20
- Apache Maven 3.9.1
- VSCode Editor 1.97.2
What is Jakarta CDI
Jakarta CDI is a feature of Jakarta EE which helps to knit together the web tier and the transactional tier of the Jakarta EE platform. Contexts and Dependency Injection (CDI) enables your objects to have their dependencies provided to them automatically, instead of creating them or receiving them as parameters. It also helps to manage the lifecycle these dependencies.
Important Services provided by CDI
- Contexts: This service enables you to bind the lifecycle and interactions of stateful components to well-defined but extensible lifecycle contexts.
- Dependency injection: This service enables you to inject components into an application in a typesafe way and to choose at deployment time which implementation of a particular interface to inject.
NOTE: This demo uses sample examples from Using the Tutorial Examples Jakarta EE 10 tutorial and customized to build and deploy to custom glassfish server installation location.
Procedure
Step1: Ensure the following Pre-requisite installed
Ensure that you have JDK, Maven and Glassfish Enterprise application server installed on your workstation.
admin@fedser:~$ javac --version
javac 17.0.13
admin@fedser:~$ mvn --version
Apache Maven 3.9.1 (Red Hat 3.9.1-3)
Download and install the Glassfish Enterprise Application server.
Ensure that the Glassfish application server is up and running.
admin@fedser:~$ asadmin start-domain
Waiting for domain1 to start .........
Waiting finished after 8,101 ms.
Successfully started the domain : domain1
domain Location: /home/admin/middleware/stack/glassfish7/glassfish/domains/domain1
Log File: /home/admin/middleware/stack/glassfish7/glassfish/domains/domain1/logs/server.log
Admin Port: 4,848
Command start-domain executed successfully.
Validate if you able to access the Admin Console page for the Glassfish Server.
URL - http://localhost:4848/common/index.jsf
Step2: Create a maven jakartaee minimal project
Here we will create a maven archetype project using the CLI with the below mentioned options to generate a minimal jakartaee version 10 project and remove the unnecessary files and folders.
admin@fedser:vscodeprojects$ mvn archetype:generate -DarchetypeArtifactId="jakartaee10-minimal" -DarchetypeGroupId="org.eclipse.starter" -DarchetypeVersion="1.1.0" -DgroupId="com.stack" -DartifactId="cdidemo"
admin@fedser:vscodeprojects$ cd cdidemo/
admin@fedser:cdidemo$ rm -rf src/main/java/com/stack/cdidemo/*
admin@fedser:cdidemo$ rm -rf src/main/resources
Step3: Create Greeting.java class
Here Greeting.java class is the CDI bean. CDI beans are classes that CDI can instantiate, manage, and inject automatically to satisfy the dependencies of other objects.
- @Dependent: The default scope if none is specified; it means that an object exists to serve exactly one client (bean) and has the same lifecycle as that client (bean).
admin@fedser:cdidemo$ cat src/main/java/com/stack/cdidemo/Greeting.java
package com.stack.cdidemo;
import jakarta.enterprise.context.Dependent;
@Dependent
public class Greeting {
public String greet(String name) {
return "Hello, " + name + ".";
}
}
Step4: Create Printer.java
Here Printer.java class is the managed bean with requestscope that deepends on the Greeting.java managed bean. We use the @Inject annotation to automatically instantiate this object and inject it here as a depdendency for this Object.
Here are the annoation and their description that we are using for the class.
- @Named: To make a bean accessible through the EL, use the @Named built-in qualifier. The @Named qualifier allows you to access the bean by using the bean name, with the first letter in lowercase. For example, a Facelets page would refer to the bean as printer.
- @RequestScoped: A user’s interaction with a web application in a single HTTP request
- @Inject: The @Inject annotation lets us define an injection point that is injected during bean instantiation
admin@fedser:cdidemo$ cat src/main/java/com/stack/cdidemo/Printer.java
package com.stack.cdidemo;
import jakarta.enterprise.context.RequestScoped;
import jakarta.inject.Inject;
import jakarta.inject.Named;
@Named
@RequestScoped
public class Printer {
@Inject
Greeting greeting;
private String name;
private String salutation;
public void createSalutation() {
this.salutation = greeting.greet(name);
}
public String getSalutation() {
return salutation;
}
public void setName(String name) {
this.name = name;
}
public String getName() {
return name;
}
}
Step5: Create CSS file
This is a customized CSS file for styling our xhtml files.
admin@fedser:cdidemo$ cat src/main/webapp/resources/css/default.css
/**
* Copyright (c), Eclipse Foundation, Inc. and its licensors.
*
* All rights reserved.
*
* This program and the accompanying materials are made available under the
* terms of the Eclipse Distribution License v1.0, which is available at
* https://www.eclipse.org/org/documents/edl-v10.php
*
* SPDX-License-Identifier: BSD-3-Clause
*/
body {
background-color: #ffffff;
font-size: 12px;
font-family: Verdana, "Verdana CE", Arial, "Arial CE", "Lucida Grande CE", lucida, "Helvetica CE", sans-serif;
color: #000000;
margin: 10px;
}
h1 {
font-family: Arial, "Arial CE", "Lucida Grande CE", lucida, "Helvetica CE", sans-serif;
border-bottom: 1px solid #AFAFAF;
font-size: 16px;
font-weight: bold;
margin: 0px;
padding: 0px;
color: #D20005;
}
a:link, a:visited {
color: #045491;
font-weight : bold;
text-decoration: none;
}
a:link:hover, a:visited:hover {
color: #045491;
font-weight : bold;
text-decoration : underline;
}
Step6: Create template.xhtml
Here is the template.xhtml. A template page is used as a template for other pages, usually referred to as client pages.
admin@fedser:cdidemo$ cat src/main/webapp/template.xhtml
<?xml version='1.0' encoding='UTF-8' ?>
<!--
Copyright (c), Eclipse Foundation, Inc. and its licensors.
All rights reserved.
This program and the accompanying materials are made available under the
terms of the Eclipse Distribution License v1.0, which is available at
https://www.eclipse.org/org/documents/edl-v10.php
SPDX-License-Identifier: BSD-3-Clause
-->
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html lang="en"
xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="jakarta.faces.html"
xmlns:ui="jakarta.faces.facelets">
<h:head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8"/>
<h:outputStylesheet library="css" name="default.css"/>
<title><ui:insert name="title">Default Title</ui:insert></title>
</h:head>
<body>
<div id="container">
<div id="header">
<h2><ui:insert name="head">Head</ui:insert></h2>
</div>
<div id="space">
<p></p>
</div>
<div id="content">
<ui:insert name="content"/>
</div>
</div>
</body>
</html>
Step7: Create index.xhtml
Here is the index.xhtml page as shown below.
admin@fedser:cdidemo$ cat src/main/webapp/index.xhtml
<?xml version='1.0' encoding='UTF-8' ?>
<!--
Copyright (c), Eclipse Foundation, Inc. and its licensors.
All rights reserved.
This program and the accompanying materials are made available under the
terms of the Eclipse Distribution License v1.0, which is available at
https://www.eclipse.org/org/documents/edl-v10.php
SPDX-License-Identifier: BSD-3-Clause
-->
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html lang="en"
xmlns="http://www.w3.org/1999/xhtml"
xmlns:ui="jakarta.faces.facelets"
xmlns:h="jakarta.faces.html">
<ui:composition template="/template.xhtml">
<ui:define name="title">Simple Greeting</ui:define>
<ui:define name="head">Simple Greeting</ui:define>
<ui:define name="content">
<h:form id="greetme">
<p><h:outputLabel value="Enter your name: " for="name"/>
<h:inputText id="name" value="#{printer.name}"/></p>
<p><h:commandButton value="Say Hello"
action="#{printer.createSalutation}"/></p>
<p><h:outputText value="#{printer.salutation}"/> </p>
</h:form>
</ui:define>
</ui:composition>
</html>
Step8: Update pom.xml
Here we are updating the pom.xml “properties” section to add “glassfish.home” directory where the glassfish server is installed. Also we are updating the plugins section with “org.codehaus.cargo” plugin to carry out automated deployment using the mvn cli to the glassfish server based on configuration properties that are provided. If you are not using this plugin you can generate the war package and deploy it manually to your glassfish server.
admin@fedser:cdidemo$ cat pom.xml
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>com.stack</groupId>
<artifactId>cdidemo</artifactId>
<version>1.0-SNAPSHOT</version>
<packaging>war</packaging>
<name>cdidemo</name>
<properties>
<maven.compiler.release>17</maven.compiler.release>
<project.build.sourceEncoding>UTF-8</project.build.sourceEncoding>
<glassfish.home>/home/admin/middleware/stack/glassfish7</glassfish.home>
</properties>
<dependencies>
<dependency>
<groupId>jakarta.platform</groupId>
<artifactId>jakarta.jakartaee-api</artifactId>
<version>10.0.0</version>
<scope>provided</scope>
</dependency>
</dependencies>
<build>
<finalName>cdidemo</finalName>
<plugins>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-compiler-plugin</artifactId>
<version>3.10.1</version>
</plugin>
<plugin>
<groupId>org.apache.maven.plugins</groupId>
<artifactId>maven-war-plugin</artifactId>
<version>3.3.2</version>
<configuration>
<failOnMissingWebXml>false</failOnMissingWebXml>
</configuration>
</plugin>
<plugin>
<groupId>org.codehaus.cargo</groupId>
<artifactId>cargo-maven3-plugin</artifactId>
<version>1.10.6</version>
<executions>
<execution>
<id>deploy</id>
<phase>integration-test</phase>
<goals>
<goal>redeploy</goal>
</goals>
</execution>
</executions>
<configuration>
<container>
<containerId>glassfish7x</containerId>
<type>installed</type>
<home>${glassfish.home}</home>
</container>
<configuration>
<type>existing</type>
<home>${glassfish.home}/glassfish/domains</home>
<properties>
<cargo.glassfish.domain.name>domain1</cargo.glassfish.domain.name>
</properties>
</configuration>
</configuration>
</plugin>
</plugins>
</build>
</project>
Step9: Create web.xml file
In a typical Jakarta Faces application, the controller (the “C” part of MVC) is registered in the Jakarta Servlet deployment descriptor file, the src/main/webapp/WEB-INF/web.xml. It basically instructs the servlet container to create an instance of jakarta.faces.webapp.FacesServlet as facesServlet during startup and to execute it when the URL pattern of the HTTP request matches *.xhtml.
admin@fedser:cdidemo$ cat src/main/webapp/WEB-INF/web.xml
<?xml version="1.0" encoding="UTF-8"?>
<!--
Copyright (c), Eclipse Foundation, Inc. and its licensors.
All rights reserved.
This program and the accompanying materials are made available under the
terms of the Eclipse Distribution License v1.0, which is available at
https://www.eclipse.org/org/documents/edl-v10.php
SPDX-License-Identifier: BSD-3-Clause
-->
<web-app version="5.0"
xmlns="https://jakarta.ee/xml/ns/jakartaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="https://jakarta.ee/xml/ns/jakartaee https://jakarta.ee/xml/ns/jakartaee/web-app_5_0.xsd">
<servlet>
<servlet-name>Faces Servlet</servlet-name>
<servlet-class>jakarta.faces.webapp.FacesServlet</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>Faces Servlet</servlet-name>
<url-pattern>*.xhtml</url-pattern>
</servlet-mapping>
<session-config>
<session-timeout>
30
</session-timeout>
</session-config>
<welcome-file-list>
<welcome-file>index.xhtml</welcome-file>
</welcome-file-list>
</web-app>
Step10: Build and Package Application
Now it’s time to build, package and deploy the application to glassfish application server as shown below.
admin@fedser:cdidemo$ mvn clean package cargo:deploy
Step11: Validate Application
We can now try to access our CDI application using the below url and validate its response.
URL - http://localhost:8080/cdidemo/


Hope you enjoyed reading this article. Thank you..
Leave a Reply
You must be logged in to post a comment.